Java中EasyExcel 合并表头注解
时间: 2024-10-22 15:28:23 浏览: 83
在Java中,EasyExcel是一个基于Apache POI和JDK流处理技术的高性能、轻量级的Excel读写库。它支持注解的方式方便地处理数据,包括合并单元格。对于合并表头,你可以使用`@TableHead`注解配合`@CellValue`注解来实现。
例如,如果你想在一个Excel文件的表头行合并A列和B列的数据,你可以这样做:
```java
@Table(name = "your_table_name", sheet = 0) // 定义表名和起始sheet
@Data // 使用Lombok等工具简化getter/setter生成
public class YourData {
@TableHead(startCol = 0, endCol = 1) // 开始列索引和结束列索引用于合并
private String headerColumn1;
@TableHead(startCol = 2, endCol = 2) // 如果B列单独显示,则单独指定
private String headerColumn2;
// 其他列...
}
```
这里,`startCol`和`endCol`属性指定了需要合并的范围。当你读取或写入Excel数据时,EasyExcel会自动解析这种注解设置,将相应的表头内容合并到一起。
相关问题
easyexcel合并单元格注解
在EasyExcel中,可以使用`@ExcelProperty`注解来声明单元格的位置和内容。如果需要合并单元格,则可以在注解中使用`@ContentRowHeight`、`@HeadRowHeight`和`@ColumnWidth`来设置合并后单元格的高度和宽度。
例如,以下是一个合并单元格的示例:
```java
public class TestDto {
@ExcelProperty(value = {"表头1", "表头1.1"}, index = 0)
@ContentRowHeight(30)
@HeadRowHeight(30)
@ColumnWidth(25)
private String col1;
@ExcelProperty(value = {"表头2", "表头2.1"}, index = 1)
@ContentRowHeight(30)
@HeadRowHeight(30)
@ColumnWidth(25)
private String col2;
@ExcelProperty(value = {"表头3", "表头3.1"}, index = 2)
@ContentRowHeight(30)
@HeadRowHeight(30)
@ColumnWidth(25)
private String col3;
@ExcelProperty(value = {"表头4", "表头4.1", "表头4.2"}, index = 3)
@ContentRowHeight(60)
@HeadRowHeight(30)
@ColumnWidth(25)
private String col4;
// 省略getter/setter方法
}
```
在以上示例中,`@ExcelProperty`注解中的`value`属性表示单元格的位置,使用数组来表示多级表头。`@ContentRowHeight`、`@HeadRowHeight`和`@ColumnWidth`注解分别表示合并后单元格的高度和宽度。其中,`@ContentRowHeight`和`@HeadRowHeight`设置的是行高,`@ColumnWidth`设置的是列宽。
以上示例中,第4列的单元格需要合并三个表头,因此在`@ExcelProperty`注解中使用了一个长度为3的数组。同时,通过`@ContentRowHeight`注解设置合并后单元格的高度为60,即占用两行。
easyexcel 实现表头合并
EasyExcel 是一个基于 Java 的 Excel 操作工具,可以用它来读取、写入 Excel 文件。在 EasyExcel 中,实现表头合并可以通过 `@ExcelProperty` 注解的 `index` 属性来实现。
假设我们有一个类 `User`,其中包含了多个属性,如下所示:
```java
public class User {
@ExcelProperty(value = "姓名", index = 0)
private String name;
@ExcelProperty(value = "年龄", index = 1)
private Integer age;
@ExcelProperty(value = "联系方式", index = 2)
private String phone;
@ExcelProperty(value = "家庭地址", index = 3)
private String address;
}
```
在上面的代码中,我们使用了 `@ExcelProperty` 注解来标识 Excel 表头的内容和位置。现在我们想要将“联系方式”和“家庭地址”这两个表头进行合并,可以将它们的 `index` 属性值设为相同的值,如下所示:
```java
public class User {
@ExcelProperty(value = "姓名", index = 0)
private String name;
@ExcelProperty(value = "年龄", index = 1)
private Integer age;
@ExcelProperty(value = {"联系方式", "家庭地址"}, index = 2)
private String phoneAndAddress;
}
```
在上面的代码中,我们使用了一个字符串数组来表示合并后的表头,其中第一个元素表示合并后的表头的名称,后面的元素表示被合并的原始表头的名称。这样,EasyExcel 就会自动将它们进行合并,并生成一个单元格。
需要注意的是,使用 `@ExcelProperty` 注解时,如果要合并表头,需要使用数组来表示表头内容,其中第一个元素表示合并后的表头的名称,后面的元素表示被合并的原始表头的名称。
阅读全文
相关推荐
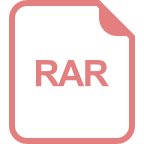
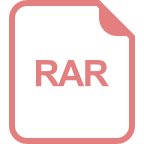
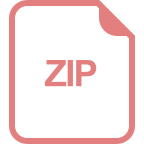












