在数组中查找元素key,返回查找到的下标,如没有找到返回-1
时间: 2024-09-20 21:13:48 浏览: 46
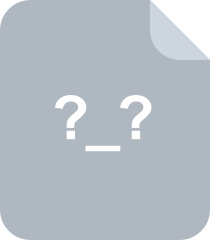
二分查找返回key在数组中的位置(下标),有注释

在数组中查找特定元素(key)并返回其索引的过程通常被称为线性搜索,因为它是从数组的第一个元素开始逐个比较,直到找到匹配项或遍历完整个数组。如果找到对应的元素,则返回该元素的索引;如果没有找到,由于数组的有序性或无序性不同,处理方式也不同:
1. **有序数组**(如升序排序的数组):二分查找效率较高。从中间元素开始,如果 key 小于中间元素,则在左半部分继续查找,反之则在右半部分。这个过程会不断缩小查找范围,直到找到 key 或确定不存在。
2. **无序数组**(未排序或随机排列):只能简单地从第一个元素逐个比对,直到找到或搜索完所有元素。这通常时间复杂度为 O(n),其中 n 为数组长度。
如果需要在 JavaScript 中实现这样的功能,可以编写如下的函数:
```javascript
function searchArray(array, key) {
for (let i = 0; i < array.length; i++) {
if (array[i] === key) {
return i;
}
}
return -1;
}
```
阅读全文
相关推荐
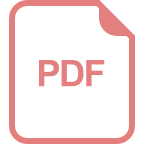
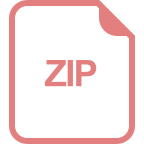















