用set怎么实现优先队列c++
时间: 2024-10-03 16:03:06 浏览: 34
在C++中,标准库并没有直接提供优先队列(Priority Queue),但是你可以通过`std::priority_queue`容器配合自定义的比较函数或适配器来实现一个简单的优先级排序的功能。`std::priority_queue`默认使用最大堆(即最小元素位于根节点),如果你想实现一个最小堆(即最大元素在根节点),可以使用`std::make_heap`、`push_heap`和`pop_heap`等函数手动管理堆。
以下是一个基本的示例,展示如何创建一个基于`int`类型的最小优先队列:
```cpp
#include <queue>
#include <vector>
// 自定义一个比较函数,用于确定元素的优先级
bool compare(const int& a, const int& b) {
return a > b; // 如果需要最大值优先,改为 a < b
}
class CustomPriorityQueue {
private:
std::vector<int> pq;
bool is_heap;
public:
void push(int value) {
pq.push(value);
if (pq.size() > 1) {
// 将最后一个元素调整到堆顶位置
std::push_heap(pq.begin(), pq.end(), compare);
is_heap = true;
}
}
int top() {
if (is_heap) {
return pq.front(); // 返回堆顶元素(当前最高优先级)
} else {
throw std::runtime_error("Priority queue is empty or not maintained as heap.");
}
}
void pop() {
if (is_heap) {
pq.pop();
if (!pq.empty()) {
// 移除堆顶元素后,重新调整堆
std::pop_heap(pq.begin(), pq.end(), compare);
pq.pop();
is_heap = pq.size() > 1;
if (is_heap) {
std::push_heap(pq.begin(), pq.end(), compare);
}
} else {
is_heap = false;
}
} else {
throw stdruntime_error("Priority queue is empty or not maintained as heap.");
}
}
};
阅读全文
相关推荐
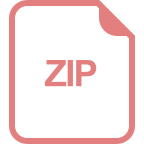
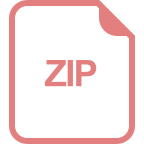
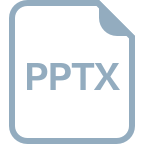
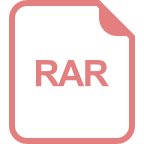
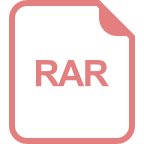
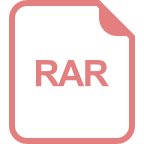
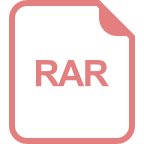
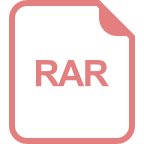
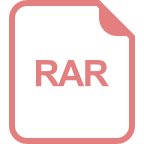
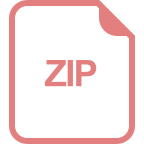
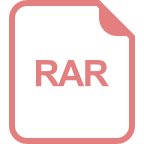
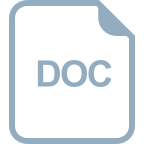
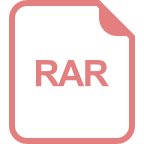
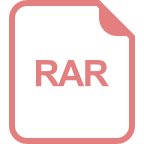
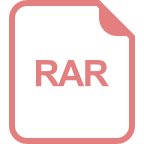
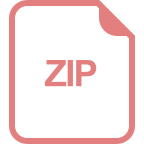
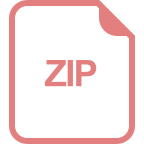
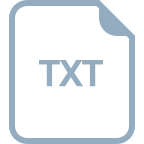
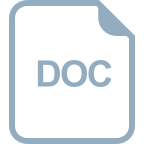