定义一个图像数组,通过24位转换为32位数据,储存在屏幕数组中,用c来简单实现
时间: 2024-10-24 09:05:30 浏览: 10
在C语言中,为了将24位颜色图像数组转换为32位(通常称为“BGR”或“ARGB”,因为32位中包含了红、绿、蓝和透明度通道),你可以创建一个新的32位像素结构体,并用循环逐个像素填充。这里是一个简单的示例:
首先,定义一个32位像素结构体:
```c
typedef struct {
unsigned char b; // Blue
unsigned char g; // Green
unsigned char r; // Red
unsigned char a; // Alpha (transparency), usually 0xFF for opaque and 0x00 for fully transparent
} Pixel32;
```
然后,假设我们有一个24位颜色的数组`img_24bit[]`,每个元素表示一个像素(例如,一个像素由8字节组成,每字节对应一个颜色分量RGB,如`img_24bit[0] = {R, G, B}`)。我们可以创建一个临时32位数组`temp_32bit`,并按需填充:
```c
void convertTo32Bit(unsigned char img_24bit[], Pixel32* temp_32bit, int width, int height) {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
// 提取24位像素
unsigned char blue = img_24bit[(y * width + x) * 3];
unsigned char green = img_24bit[(y * width + x) * 3 + 1];
unsigned char red = img_24bit[(y * width + x) * 3 + 2];
// 填充到32位像素结构
temp_32bit[y * width + x].b = blue;
temp_32bit[y * width + x].g = green;
temp_32bit[y * width + x].r = red;
temp_32bit[y * width + x].a = 0xff; // 假设全透明
}
}
}
```
最后,你需要确保有足够的内存空间来存储`temp_32bit`数组。
阅读全文
相关推荐
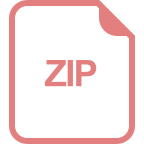
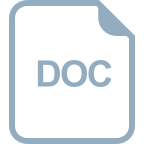
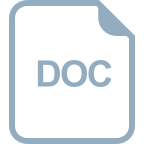
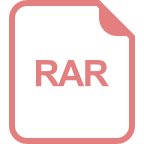
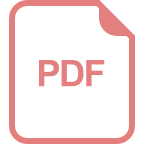
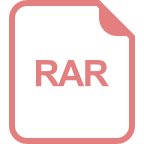
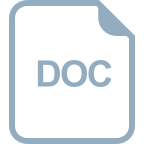
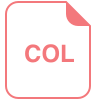
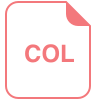
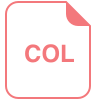
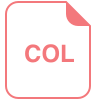


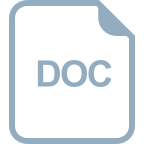
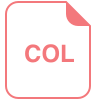



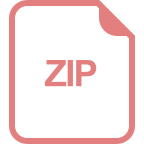