将numpy数组转换为图像并存储在指定文件夹下
时间: 2023-12-23 20:05:39 浏览: 32
要将numpy数组转换为图像并存储在指定文件夹下,可以使用Python中的PIL库。下面是一个示例代码:
```python
from PIL import Image
import numpy as np
import os
# 创建一个numpy数组
arr = np.random.rand(100, 100, 3) * 255
arr = arr.astype('uint8')
# 创建一个图像对象
img = Image.fromarray(arr)
# 定义要保存图像的文件夹路径和文件名
folder_path = 'path/to/folder'
file_name = 'image.jpg'
# 确保文件夹存在
if not os.path.exists(folder_path):
os.makedirs(folder_path)
# 将图像保存到指定文件夹下
img.save(os.path.join(folder_path, file_name))
```
这个代码将创建一个大小为100x100,像素值在0-255之间的随机的RGB彩色图像,然后将其保存为JPEG文件格式在指定的文件夹中。如果需要保存为其他格式的图像,只需更改文件名的扩展名即可。
相关问题
加载训练集(图像在trainvol中,为Png格式,怎么操作
加载训练集需要先将PNG格式的图像数据读入到Python中,然后将其转换为Numpy数组格式,最后将所有图像数据存储在一个Numpy数组中。以下是基本步骤的示例代码:
```python
import os
import numpy as np
from PIL import Image
# 设置图像目录路径
train_dir = './trainvol'
# 获取目录中的所有文件名
train_files = os.listdir(train_dir)
# 初始化存储图像数据的数组
train_data = np.zeros((len(train_files), height, width, channels), dtype=np.uint8)
# 逐个读取图像数据并存储到Numpy数组中
for i, file in enumerate(train_files):
# 读取图像数据并转换为Numpy数组格式
img = Image.open(os.path.join(train_dir, file))
img = np.array(img)
# 存储图像数据到数组中
train_data[i] = img
# 存储训练数据到Numpy文件中
np.save('train_data.npy', train_data)
```
其中,`train_dir`是训练集图像所在的文件夹路径,`train_files`是该文件夹中所有图像文件的文件名列表,`train_data`是存储所有图像数据的Numpy数组,`height`、`width`和`channels`是图像的高度、宽度和通道数。在示例代码中,我们使用了Python的内置库`os`来获取目录中的所有文件名,使用`PIL`库中的`Image`函数来读取PNG格式的图像数据。最后,我们将所有图像数据存储到一个Numpy数组中,并使用`np.save()`函数将其存储到Numpy二进制文件中。
trainseg是有标签图像的普通文件夹,怎么加载这个训练集标签
如果`trainseg`是一个有标签图像的普通文件夹,我们可以使用类似的方法读取图像数据和标签数据,并将它们存储到Numpy数组中。以下是基本步骤的示例代码:
```python
import os
import numpy as np
from PIL import Image
# 设置图像和标签目录路径
train_dir = './trainvol'
label_dir = './trainseg'
# 获取目录中的所有文件名
train_files = os.listdir(train_dir)
label_files = os.listdir(label_dir)
# 初始化存储图像和标签数据的数组
train_data = np.zeros((len(train_files), height, width, channels), dtype=np.uint8)
label_data = np.zeros((len(label_files), height, width), dtype=np.uint8)
# 逐个读取图像和标签数据并存储到Numpy数组中
for i, file in enumerate(train_files):
# 读取图像数据并转换为Numpy数组格式
img = Image.open(os.path.join(train_dir, file))
img = np.array(img)
# 存储图像数据到数组中
train_data[i] = img
# 读取标签数据并转换为Numpy数组格式
label = Image.open(os.path.join(label_dir, label_files[i]))
label = np.array(label)
# 存储标签数据到数组中
label_data[i] = label
# 存储训练数据和标签数据到Numpy文件中
np.save('train_data.npy', train_data)
np.save('train_labels.npy', label_data)
```
其中,`train_dir`是训练集图像所在的文件夹路径,`label_dir`是训练集标签所在的文件夹路径,`train_files`和`label_files`分别是两个目录中所有文件的文件名列表,`train_data`和`label_data`分别是存储所有图像数据和标签数据的Numpy数组,`height`、`width`和`channels`是图像的高度、宽度和通道数。在示例代码中,我们使用了Python的内置库`os`来获取目录中的所有文件名,使用`PIL`库中的`Image`函数来读取PNG格式的图像数据。最后,我们将所有图像数据和标签数据存储到各自的Numpy数组中,并使用`np.save()`函数将其存储到Numpy二进制文件中。
相关推荐
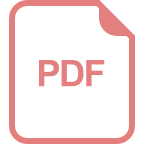
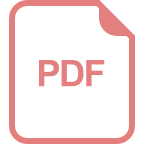












