c++memset库文件
时间: 2023-10-21 21:25:19 浏览: 93
C语言中的memset函数是一个库函数,位于<string.h>头文件中。它用于将一块内存区域的每个字节都设置为特定的值。
函数原型如下:
void *memset(void *ptr, int value, size_t num);
参数说明:
- ptr:指向要填充的内存区域的指针。
- value:要设置的值,以int类型表示。
- num:要设置的字节数。
函数返回一个指向目标内存区域的指针。它常用于对数组、结构体等内存块进行初始化或清零操作。
例如,下面的代码将一个整型数组清零:
#include <stdio.h>
#include <string.h>
int main() {
int arr;
memset(arr, 0, sizeof(arr)); // 将arr数组的每个字节都设置为0
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]); // 输出:0 0 0 0 0
}
return 0;
}
请注意,memset函数操作的是字节,而不是具体的数据类型。所以当填充非字符类型数据时,需要先将要设置的值转换为对应字节表示。
相关问题
c++ socket发文件
在C语言中,可以使用socket库中的函数来发送文件。下面是一个简单的示例代码,可以将一个文件发送到服务器。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#define PORT 8888
int main(int argc, char const *argv[])
{
int sock = 0, valread;
struct sockaddr_in serv_addr;
char buffer[1024] = {0};
// 创建套接字
if ((sock = socket(AF_INET, SOCK_STREAM, 0)) < 0)
{
printf("\n Socket creation error \n");
return -1;
}
// 设置服务器地址和端口
memset(&serv_addr, '0', sizeof(serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(PORT);
// 将IP地址从字符串转换为网络字节序
if(inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr)<=0)
{
printf("\nInvalid address/ Address not supported \n");
return -1;
}
// 连接到服务器
if (connect(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0)
{
printf("\nConnection Failed \n");
return -1;
}
// 打开文件
FILE *fp = fopen("test.txt", "rb");
if (fp == NULL) {
printf("File open error\n");
return -1;
}
// 读取文件内容并发送
int file_size = 0;
while ((file_size = fread(buffer, sizeof(char), 1024, fp)) > 0) {
if (send(sock, buffer, file_size, 0) != file_size) {
printf("Send error\n");
return -1;
}
memset(buffer, 0, 1024);
}
// 关闭文件和套接字
fclose(fp);
close(sock);
return 0;
}
```
该代码将一个名为test.txt的文件发送到IP地址为127.0.0.1,端口号为8888的服务器。在实际应用中,需要根据实际情况修改IP地址和端口号,并对发送和接收数据的代码进行适当的修改。
c++ zlib库的使用
zlib是一个用于压缩和解压缩数据的开源库,它常于将文件或网络传输的数据进行压缩,以减少传输时间和网络流量。
下面是使用zlib库进行压缩和解压缩的示例代码:
压缩:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <cstring>
#include "zlib.h"
bool compress(const std::string& in_file, const std::string& out_file)
{
std::ifstream fin(in_file, std::ios::binary);
if (!fin) {
std::cerr << "Failed to open input file: " << in_file << std::endl;
return false;
}
std::ofstream fout(out_file, std::ios::binary);
if (!fout) {
std::cerr << "Failed to open output file: " << out_file << std::endl;
return false;
}
const int BUF_SIZE = 1024 * 8;
char in_buf[BUF_SIZE];
char out_buf[BUF_SIZE];
z_stream zs;
memset(&zs, 0, sizeof(zs));
if (deflateInit(&zs, Z_DEFAULT_COMPRESSION) != Z_OK) {
std::cerr << "Failed to initialize zlib compression" << std::endl;
return false;
}
while (true) {
fin.read(in_buf, BUF_SIZE);
std::streamsize bytes_in = fin.gcount();
if (bytes_in == 0) {
break;
}
zs.next_in = reinterpret_cast<Bytef*>(in_buf);
zs.avail_in = static_cast<uInt>(bytes_in);
do {
zs.next_out = reinterpret_cast<Bytef*>(out_buf);
zs.avail_out = BUF_SIZE;
int ret = deflate(&zs, Z_FINISH);
if (ret == Z_STREAM_ERROR) {
std::cerr << "Failed to compress input data" << std::endl;
deflateEnd(&zs);
return false;
}
std::streamsize bytes_out = BUF_SIZE - zs.avail_out;
fout.write(out_buf, bytes_out);
} while (zs.avail_out == 0);
}
deflateEnd(&zs);
return true;
}
```
解压缩:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <cstring>
#include "zlib.h"
bool decompress(const std::string& in_file, const std::string& out_file)
{
std::ifstream fin(in_file, std::ios::binary);
if (!fin) {
std::cerr << "Failed to open input file: " << in_file << std::endl;
return false;
}
std::ofstream fout(out_file, std::ios::binary);
if (!fout) {
std::cerr << "Failed to open output file: " << out_file << std::endl;
return false;
}
const int BUF_SIZE = 1024 * 8;
char in_buf[BUF_SIZE];
char out_buf[BUF_SIZE];
z_stream zs;
memset(&zs, 0, sizeof(zs));
if (inflateInit(&zs) != Z_OK) {
std::cerr << "Failed to initialize zlib decompression" << std::endl;
return false;
}
while (true) {
fin.read(in_buf, BUF_SIZE);
std::streamsize bytes_in = fin.gcount();
if (bytes_in == 0) {
break;
}
zs.next_in = reinterpret_cast<Bytef*>(in_buf);
zs.avail_in = static_cast<uInt>(bytes_in);
do {
zs.next_out = reinterpret_cast<Bytef*>(out_buf);
zs.avail_out = BUF_SIZE;
int ret = inflate(&zs, Z_NO_FLUSH);
if (ret == Z_STREAM_ERROR) {
std::cerr << "Failed to decompress input data" << std::endl;
inflateEnd(&zs);
return false;
}
std::streamsize bytes_out = BUF_SIZE - zs.avail_out;
fout.write(out_buf, bytes_out);
} while (zs.avail_out == 0);
}
inflateEnd(&zs);
return true;
}
```
在上述代码中,使用了z_stream结构体来保存压缩或解压缩的状态信息,其中deflateInit和inflateInit函数用于初始化z_stream结构体,deflate和inflate函数用于执行压缩和解压缩操作,deflateEnd和inflateEnd函数用于释放相关资源。
需要注意的是,压缩和解压缩的数据均应该是二进制数据,因此在打开文件时需要指定std::ios::binary标志。
阅读全文
相关推荐
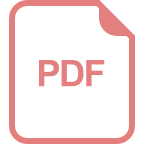
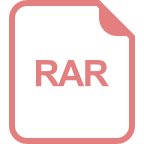
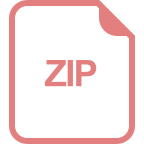
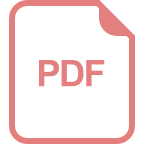
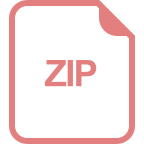
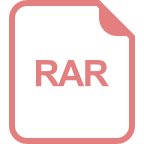
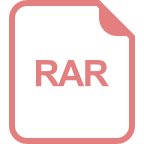
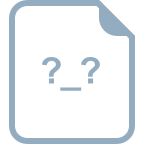
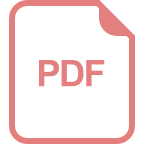
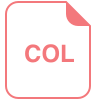




