我需要解决【TSP旅行商】问题,请列出【2】个可以用【Java】编程的优化路径算法,w展开写出这【2】个算法的编程难度级别和时间复杂度,罗列出每个算法编程要用到的库。
时间: 2024-11-09 09:17:28 浏览: 32
TSP(Traveling Salesman Problem)问题是计算机科学中的经典组合优化问题,它要求找到一条从给定城市列表出发,经过所有城市恰好一次后返回起点的最短路径。以下是两种常用的优化路径算法:
1. **贪心算法** - Nearest Neighbor (NN) 或 Farthest Insertion (FI)
- **难度级别**:入门级,适合初学者了解基本思路。
- **时间复杂度**:O(n^2),其中n是城市的数量。因为对于每个未访问的城市,都需要查找当前最优路径下最近或最远的城市。
- **库依赖**:基本的Java集合框架(如ArrayList、HashMap等)即可,无需额外库。
```java
import java.util.*;
public class TSP {
public int nearestNeighbor(int[][] distances) {
// 初始化和存储路径信息
List<Integer> path = new ArrayList<>();
int currentCity = 0;
path.add(currentCity);
for (int i = 1; i < distances.length; i++) {
int nearest = findNearest(currentCity, distances);
path.add(nearest);
currentCity = nearest;
}
// 添加回程到起点
path.add(0); // or path.add(currentCity);
return computeTotalDistance(distances, path);
}
private int findNearest(int city, int[][] distances) {
// 找到当前位置到其他城市的最小距离
int minDistance = Integer.MAX_VALUE;
int nearestCity = -1;
for (int i = 0; i < distances[city].length; i++) {
if (distances[city][i] < minDistance && i != city) {
minDistance = distances[city][i];
nearestCity = i;
}
}
return nearestCity;
}
private int computeTotalDistance(int[][] distances, List<Integer> path) {
int totalDistance = 0;
for (int i = 0; i < path.size() - 1; i++) {
totalDistance += distances[path.get(i)][path.get(i + 1)];
}
// 计算回程距离(如果尚未计算)
totalDistance += distances[path.get(path.size() - 1)][path.get(0)];
return totalDistance;
}
}
```
2. **遗传算法** (Genetic Algorithm, GA)
- **难度级别**:中级,涉及进化策略和概率论,对理解算法原理和随机过程有较高要求。
- **时间复杂度**:没有固定的表达式,GA通常迭代多次,每次迭代的时间取决于种群大小、交叉和变异操作的数量等因素。平均情况下可能接近O(n^2 * iter),其中iter是迭代次数。
- **库依赖**:可能需要使用专门的遗传算法库,例如Jung、DEAP或OptaPlanner。这些库提供了高级接口用于定义基因编码、适应度函数等。
```java
import org.jung.graph.*;
import net.sf.jung.algorithms.tsp.*;
public class GeneticAlgorithmTSP {
public int geneticAlgorithm(int[][] distances, int populationSize, int generations) {
// 使用TSPSolver或类似算法实例化遗传算法
TSPSolver solver = new HillClimbingTSPSolver();
Population<TSPSolution> population = new SimplePopulation<>(populationSize);
// 初始化随机解并计算初始适应度
for (int i = 0; i < populationSize; i++) {
population.set(i, new RandomTSPInstance(distances).generate());
}
// 进行遗传算法迭代
for (int gen = 0; gen < generations; gen++) {
population.evolve(solver);
}
// 返回最佳解的总距离
return population.getBest().getLength();
}
}
```
阅读全文
相关推荐
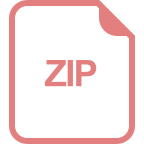
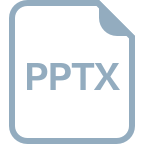





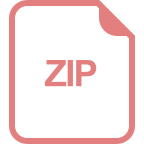
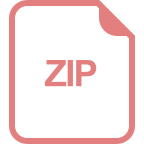
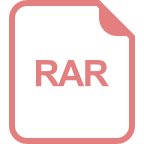
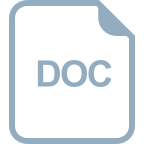
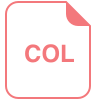
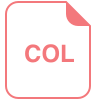
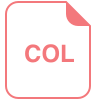
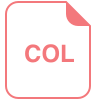
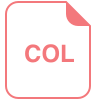
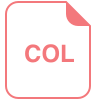
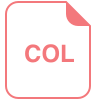