用c语言实现牛顿插值算法
时间: 2023-11-19 08:07:16 浏览: 272
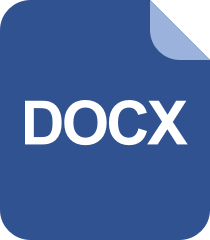
牛顿插值算法的C语言实现

牛顿插值算法是一种用于插值的数值分析方法,可以用于构造一个多项式函数,使得该函数在给定的数据点上与已知函数相等。下面是用C语言实现牛顿插值算法的代码:
```
#include <stdio.h>
// 计算差商
float calc_diff_quotient(float x[], float y[], int n, int i, int j) {
if (j == i) {
return y[i];
} else {
return (calc_diff_quotient(x, y, n, i + 1, j) - calc_diff_quotient(x, y, n, i, j - 1)) / (x[j] - x[i]);
}
}
// 计算插值多项式的值
float calc_newton_interpolation(float x[], float y[], int n, float t) {
float result = 0;
for (int i = 0; i < n; i++) {
float temp = calc_diff_quotient(x, y, n, 0, i);
for (int j = 0; j < i; j++) {
temp *= (t - x[j]);
}
result += temp;
}
return result;
}
int main() {
float x[] = {1, 2, 3, 4, 5};
float y[] = {1, 4, 9, 16, 25};
int n = 5;
float t = 2.5;
float result = calc_newton_interpolation(x, y, n, t);
printf("f(%f) = %f\n", t, result);
return 0;
}
```
上述代码中,`calc_diff_quotient`函数用于计算差商,`calc_newton_interpolation`函数用于计算插值多项式的值。在主函数中,我们定义了一组数据点,然后计算在x=2.5处的插值多项式的值。
阅读全文
相关推荐
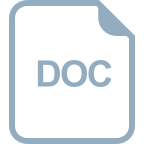
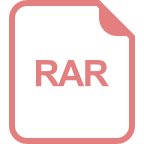
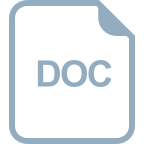
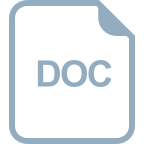

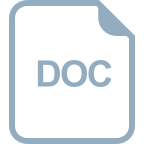

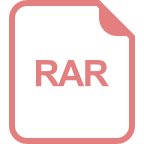
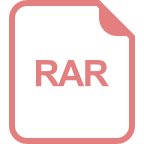
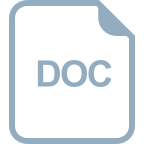
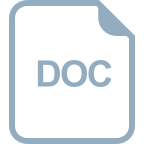
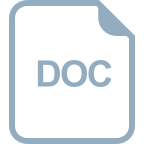
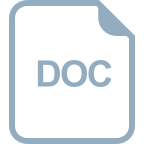
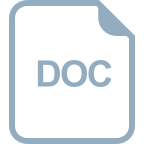
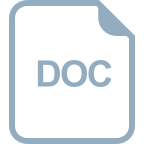