list转str以空格隔开
时间: 2023-09-08 21:02:00 浏览: 202
要将列表转换为以空格分隔的字符串,可以使用Python中的join函数。join函数是一个字符串方法,可以将序列中的字符串连接起来,以指定的分隔符作为分隔符。
以下是一个示例代码:
```python
my_list = ['apple', 'banana', 'orange']
my_str = ' '.join(my_list)
print(my_str)
```
输出结果为:
```
apple banana orange
```
在这个例子中,我们有一个包含三个水果名称的列表`my_list`。然后,我们使用join函数将列表中的元素连接成一个字符串。在join函数中,我们使用空格作为分隔符。最后,我们将结果存储在变量`my_str`中,并打印输出。
这样,我们就成功地将列表转换为以空格分隔的字符串。
相关问题
list转string 空格隔开
要将列表转换为以空格隔开的字符串,可以使用Python中的join()函数。该函数可以将列表中的元素连接起来,并用指定的分隔符分隔它们。
以下是一种方法来实现这个目标:
1. 首先,我们需要将列表中的元素转换为字符串类型。可以使用map()函数和str()函数来实现这一点。map()函数将会遍历列表中的每个元素,并将其传递给str()函数,将其转换为字符串类型。
2. 接下来,我们使用join()函数来连接转换后的字符串元素,并用空格作为分隔符。
3. 最后,我们可以将得到的字符串输出或者进行其他操作。
下面是一个示例代码:
```
my_list = ['A', 'B', 'C', 'D', 'E', 'F', 'G']
result = ' '.join(map(str, my_list))
print(result)
```
输出结果为:
```
A B C D E F G
```
在这个例子中,我们将列表`my_list`中的元素转换为字符串,并且使用join()函数将它们连接起来,用空格作为分隔符。最后,我们将结果打印出来。
请注意,这个方法只是其中一种实现列表转换为以空格隔开的字符串的方法。还有其他的方法,但这是一种简单且常用的方法。
python 输入两个数,中间以空格隔开
要在 Python 中输入两个数并以空格分隔,可以使用 `input()` 函数获取用户的输入。然后,可以使用 `split()` 函数将输入的字符串分割成一个列表,其中每个元素是以空格分隔的数值。
下面是一个示例代码:
```python
input_str = input("请输入两个数,中间以空格隔开:")
num_list = input_str.split()
# 将字符串转换为整数
num1 = int(num_list[0])
num2 = int(num_list[1])
print("第一个数:", num1)
print("第二个数:", num2)
```
在这个例子中,我们使用 `input()` 函数获取用户输入的字符串,并将其存储在 `input_str` 变量中。然后,我们使用 `split()` 函数将字符串分割成一个列表,存储在 `num_list` 变量中。接下来,我们通过索引访问列表中的元素,并使用 `int()` 函数将其转换为整数。最后,我们打印输出这两个数值。
请确保输入的字符串符合预期的格式,即两个数值之间用空格分隔。
相关推荐
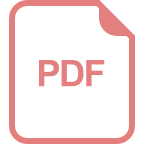
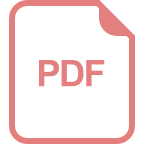
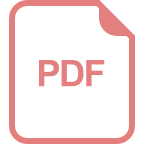













