nrf52840用keil5实现按下按键1、2、3、4,LED灯1、2、3、4分别以500ms、1s、2s、3s的时间做流水灯操作(使用定时器控制时间)
时间: 2023-12-28 11:23:22 浏览: 36
以下是nrf52840用keil5实现按下按键1、2、3、4,LED灯1、2、3、4分别以500ms、1s、2s、3s的时间做流水灯操作的代码实现:
```c
#include "nrf.h"
#include "nrf_gpio.h"
#include "nrf_delay.h"
#include "nrfx_timer.h"
#define LED1 17
#define LED2 18
#define LED3 19
#define LED4 20
#define BUTTON1 13
#define BUTTON2 14
#define BUTTON3 15
#define BUTTON4 16
nrfx_timer_t My_Timer0 = NRFX_TIMER_INSTANCE(0);
void My_Timer0_Interrupt(nrf_timer_event_t event_type, void * p_context) //1ms
{
static uint16_t flag_500ms, flag_1s, flag_2s, flag_3s;
switch (event_type)
{
case NRF_TIMER_EVENT_COMPARE0: // 匹配到了1ms的次数了
if (++flag_500ms == 500) //500ms
{
nrf_gpio_pin_toggle(LED1);
flag_500ms = 0;
}
if (++flag_1s == 1000) //1s
{
nrf_gpio_pin_toggle(LED2);
flag_1s = 0;
}
if (++flag_2s == 2000) //2s
{
nrf_gpio_pin_toggle(LED3);
flag_2s = 0;
}
if (++flag_3s == 3000) //3s
{
nrf_gpio_pin_toggle(LED4);
flag_3s = 0;
}
break;
default:
//Do nothing.
break;
}
}
int main(void)
{
nrf_gpio_cfg_output(LED1);
nrf_gpio_cfg_output(LED2);
nrf_gpio_cfg_output(LED3);
nrf_gpio_cfg_output(LED4);
nrfx_timer_config_t timer_cfg = NRFX_TIMER_DEFAULT_CONFIG;
nrfx_timer_init(&My_Timer0, &timer_cfg, My_Timer0_Interrupt);
uint32_t time_ticks;
time_ticks = nrfx_timer_ms_to_ticks(&My_Timer0, 1); //算出1ms需要计数多少次
nrfx_timer_extended_compare(&My_Timer0, NRF_TIMER_CC_CHANNEL0, time_ticks, NRF_TIMER_SHORT_COMPARE0_CLEAR_MASK, true);
nrfx_timer_enable(&My_Timer0);
while (true)
{
if (!nrf_gpio_pin_read(BUTTON1))
{
nrf_delay_ms(50);
if (!nrf_gpio_pin_read(BUTTON1))
{
nrfx_timer_pause(&My_Timer0);
nrf_gpio_pin_clear(LED1);
nrf_gpio_pin_clear(LED2);
nrf_gpio_pin_clear(LED3);
nrf_gpio_pin_clear(LED4);
nrfx_timer_clear(&My_Timer0);
nrfx_timer_resume(&My_Timer0);
}
}
if (!nrf_gpio_pin_read(BUTTON2))
{
nrf_delay_ms(50);
if (!nrf_gpio_pin_read(BUTTON2))
{
nrfx_timer_pause(&My_Timer0);
nrf_gpio_pin_clear(LED1);
nrf_gpio_pin_clear(LED2);
nrf_gpio_pin_clear(LED3);
nrf_gpio_pin_clear(LED4);
time_ticks = nrfx_timer_ms_to_ticks(&My_Timer0, 500);
nrfx_timer_extended_compare(&My_Timer0, NRF_TIMER_CC_CHANNEL0, time_ticks, NRF_TIMER_SHORT_COMPARE0_CLEAR_MASK, true);
nrfx_timer_resume(&My_Timer0);
}
}
if (!nrf_gpio_pin_read(BUTTON3))
{
nrf_delay_ms(50);
if (!nrf_gpio_pin_read(BUTTON3))
{
nrfx_timer_pause(&My_Timer0);
nrf_gpio_pin_clear(LED1);
nrf_gpio_pin_clear(LED2);
nrf_gpio_pin_clear(LED3);
nrf_gpio_pin_clear(LED4);
time_ticks = nrfx_timer_ms_to_ticks(&My_Timer0, 1000);
nrfx_timer_extended_compare(&My_Timer0, NRF_TIMER_CC_CHANNEL0, time_ticks, NRF_TIMER_SHORT_COMPARE0_CLEAR_MASK, true);
nrfx_timer_resume(&My_Timer0);
}
}
if (!nrf_gpio_pin_read(BUTTON4))
{
nrf_delay_ms(50);
if (!nrf_gpio_pin_read(BUTTON4))
{
nrfx_timer_pause(&My_Timer0);
nrf_gpio_pin_clear(LED1);
nrf_gpio_pin_clear(LED2);
nrf_gpio_pin_clear(LED3);
nrf_gpio_pin_clear(LED4);
time_ticks = nrfx_timer_ms_to_ticks(&My_Timer0, 2000);
nrfx_timer_extended_compare(&My_Timer0, NRF_TIMER_CC_CHANNEL0, time_ticks, NRF_TIMER_SHORT_COMPARE0_CLEAR_MASK, true);
nrfx_timer_resume(&My_Timer0);
}
}
}
}
```
相关推荐
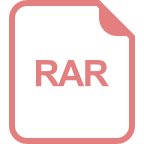
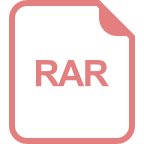














