SRS webrtc python
时间: 2023-09-13 10:11:47 浏览: 100
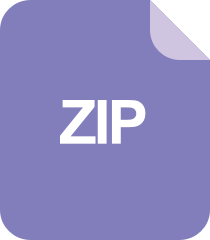
webrtc
WebRTC (Web Real-Time Communication) is a free, open-source project that enables real-time communication between web browsers, mobile applications, and IoT devices. It provides protocols and APIs for building applications such as voice and video calls, file sharing, and real-time messaging.
To implement WebRTC in Python, you can use the aiortc library, which is an asyncio-based framework for WebRTC. Here's a brief overview of how you can get started:
1. Install aiortc using pip:
```
pip install aiortc
```
2. Import the necessary modules in your Python code:
```python
import asyncio
from aiortc import RTCPeerConnection, RTCSessionDescription
```
3. Create an asyncio event loop and define your signaling logic:
```python
async def offer():
# Create a peer connection
pc = RTCPeerConnection()
# Create an offer
offer = await pc.createOffer()
await pc.setLocalDescription(offer)
# Implement your signaling to send the offer to the remote peer
async def answer(offer):
# Create a peer connection
pc = RTCPeerConnection()
# Set the remote description from the offer
await pc.setRemoteDescription(RTCSessionDescription(offer["sdp"], "offer"))
# Create an answer
answer = await pc.createAnswer()
await pc.setLocalDescription(answer)
# Implement your signaling to send the answer back to the remote peer
async def connect(answer):
# Set the remote description from the answer
await pc.setRemoteDescription(RTCSessionDescription(answer["sdp"], "answer"))
# Implement your signaling to finalize the connection
# Run the event loop
loop = asyncio.get_event_loop()
loop.run_until_complete(offer())
```
Keep in mind that this is just a basic example to get you started with WebRTC in Python using aiortc. You'll need to implement the signaling logic to exchange SDP offers and answers between peers.
For more advanced usage and additional features, refer to the aiortc documentation: https://aiortc.readthedocs.io/
Remember, WebRTC involves more than just Python code, as it requires signaling servers and potentially STUN/TURN servers for NAT traversal.
阅读全文
相关推荐
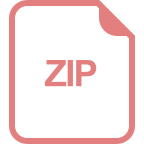
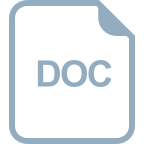

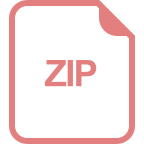
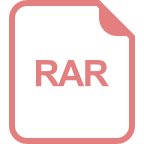
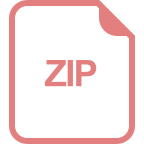







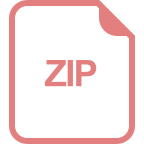