poi5.2.3 maven
时间: 2023-07-31 22:06:38 浏览: 155
对于 Poi 5.2.3 版本的 Maven 配置,您可以在项目的 pom.xml 文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.3</version>
</dependency>
```
这将导入 Poi 的核心库。如果您还需要使用 Poi 的其他模块,您可以根据需要添加相应的依赖项。例如,如果您需要使用 Poi 的 Excel 模块,可以添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
```
请确保您的项目已正确配置 Maven,并且能够从中央仓库下载依赖项。在添加依赖项后,Maven 将自动下载所需的 Poi JAR 文件并将其包含在项目构建中。
相关问题
org.apache.poi.ss.formula.functions的maven坐标
`org.apache.poi.ss.formula.functions` 是Apache POI库中用于处理Excel电子表格中公式计算的一个包。Apache POI是一个Java库,用于读取和写入Microsoft Office格式的文件,包括Excel、Word等。在Maven项目中,要使用Apache POI的这个特定包,你需要添加相关的Maven依赖到你的`pom.xml`文件中。
`org.apache.poi.ss.formula.functions` 包是Apache POI的Spreadsheet公式引擎的一部分,它并不单独作为一个依赖项存在,因此你不需要指定`org.apache.poi.ss.formula.functions`的坐标,而是应该添加整个Apache POI库的依赖。
以下是一个示例的Maven依赖配置,用于添加Apache POI库到项目中:
```xml
<dependencies>
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.3</version> <!-- 使用最新的版本号 -->
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version> <!-- 使用最新的版本号 -->
</dependency>
<!-- 其他依赖项... -->
</dependencies>
```
请注意,版本号`5.2.3`只是一个示例,你应该使用实际的最新版本号,可以查看Apache POI在Maven中央仓库的最新版本。
使用JAVA apachepoi根据该模板生成完整的代码
要使用Apache POI 根据提供的模板 `template.docx` 生成一个完整的Word文档,可以按照以下步骤编写Java代码:
### 步骤 1: 添加依赖
首先,在你的Maven项目的 `pom.xml` 文件中添加 Apache POI 的依赖:
```xml
<dependencies>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml-schemas</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.xmlbeans</groupId>
<artifactId>xmlbeans</artifactId>
<version>5.1.1</version>
</dependency>
</dependencies>
```
### 步骤 2: 编写 Java 代码
```java
import org.apache.poi.xwpf.usermodel.*;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
public class WordReportGenerator {
public static void main(String[] args) {
String templatePath = "path/to/template.docx";
String outputPath = "path/to/output.docx";
try (FileInputStream fis = new FileInputStream(templatePath);
XWPFDocument document = new XWPFDocument(fis);
FileOutputStream fos = new FileOutputStream(outputPath)) {
// 替换文本书签
replaceTextBookmarks(document, "${reportName}", "示例报告");
replaceTextBookmarks(document, "${name}", "元器件A");
replaceTextBookmarks(document, "${type}", "类型X");
replaceTextBookmarks(document, "${Color}", "红色");
replaceTextBookmarks(document, "${age}", "张三");
replaceTextBookmarks(document, "${sex}", "男");
// 替换模型需求条目
List<String> items = List.of("需求1", "需求2", "需求3");
replaceItems(document, items);
// 替换模型量化指标
List<String[]> requirementList = List.of(
new String[]{"指标1", "值1"},
new String[]{"指标2", "值2"}
);
replaceTable(document, "${requirementList}", requirementList);
// 替换模型可视化图
addImage(document, "${image}", "path/to/image.png");
// 替换项目规划图
addImage(document, "${image1}", "path/to/project_plan.png");
// 替换元器件功能指标
List<String[]> requirementList1 = List.of(
new String[]{"功能1", "描述1"},
new String[]{"功能2", "描述2"}
);
replaceTable(document, "${requirementList1}", requirementList1);
// 替换元器件性能指标
List<String[]> requirementList2 = List.of(
new String[]{"性能1", "值1"},
new String[]{"性能2", "值2"}
);
replaceTable(document, "${requirementList2}", requirementList2);
// 替换元器件环境指标
List<String[]> requirementList3 = List.of(
new String[]{"环境1", "值1"},
new String[]{"环境2", "值2"}
);
replaceTable(document, "${requirementList3}", requirementList3);
// 替换元器件可靠性指标
List<String[]> requirementList4 = List.of(
new String[]{"可靠性1", "值1"},
new String[]{"可靠性2", "值2"}
);
replaceTable(document, "${requirementList4}", requirementList4);
// 替换元器件内部模块图
addImage(document, "${image2}", "path/to/module_diagram.png");
// 保存文档
document.write(fos);
} catch (IOException e) {
e.printStackTrace();
}
}
private static void replaceTextBookmarks(XWPFDocument document, String placeholder, String replacement) {
for (XWPFParagraph paragraph : document.getParagraphs()) {
if (paragraph.getText().contains(placeholder)) {
paragraph.getRuns().forEach(run -> run.setText(run.getText(0).replace(placeholder, replacement), 0));
}
}
}
private static void replaceItems(XWPFDocument document, List<String> items) {
StringBuilder sb = new StringBuilder();
int index = 1;
for (String item : items) {
sb.append(index).append("、 ").append(item).append("\n");
index++;
}
replaceTextBookmarks(document, "${items}", sb.toString());
}
private static void replaceTable(XWPFDocument document, String placeholder, List<String[]> data) throws IOException {
for (XWPFTable table : document.getTables()) {
for (XWPFTableRow row : table.getRows()) {
for (XWPFTableCell cell : row.getCells()) {
if (cell.getText().contains(placeholder)) {
cell.removeParagraph(cell.getParagraphs().get(0));
for (String[] rowData : data) {
XWPFParagraph paragraph = cell.addParagraph();
for (String value : rowData) {
paragraph.createRun().setText(value + "\t");
}
}
}
}
}
}
}
private static void addImage(XWPFDocument document, String placeholder, String imagePath) throws IOException {
for (XWPFParagraph paragraph : document.getParagraphs()) {
if (paragraph.getText().contains(placeholder)) {
int pictureIndex = document.getPicturesData().size();
byte[] bytes = new byte[0];
try (FileInputStream fis = new FileInputStream(imagePath)) {
bytes = fis.readAllBytes();
}
XWPFPictureData pictureData = document.addPictureData(bytes, Document.PICTURE_TYPE_PNG);
document.getAllPictures().add(pictureData);
XWPFRun run = paragraph.createRun();
run.addPicture(pictureData, Document.PICTURE_TYPE_PNG, imagePath, Units.toEMU(200), Units.toEMU(200));
paragraph.getRuns().forEach(r -> r.setText(r.getText(0).replace(placeholder, ""), 0));
}
}
}
}
```
### 解释
1. **添加依赖**: 在 `pom.xml` 中添加 Apache POI 的依赖。
2. **读取模板文件**: 使用 `FileInputStream` 读取模板文件,并创建 `XWPFDocument` 对象。
3. **替换文本书签**: 遍历文档中的所有段落,找到包含占位符的段落并进行替换。
4. **替换模型需求条目**: 将需求条目列表转换为字符串,并替换相应的占位符。
5. **替换表格**: 找到包含占位符的表格单元格,并用新的数据行替换。
6. **插入图片**: 找到包含占位符的段落,插入图片并删除占位符。
7. **保存文档**: 将修改后的文档保存到指定路径。
通过以上步骤,你可以根据模板生成一个完整的Word文档。
阅读全文
相关推荐
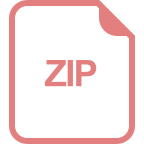
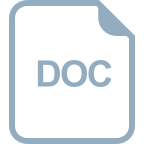
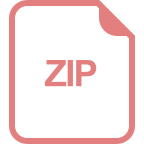
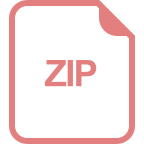
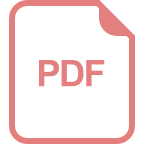
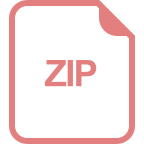
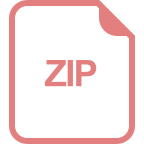
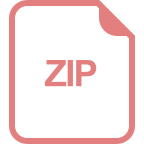
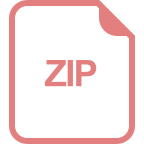
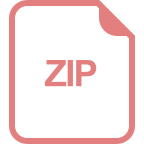
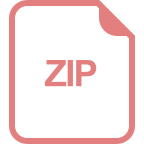
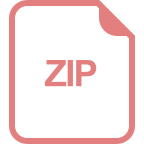
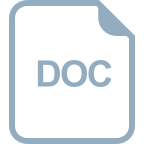
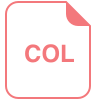
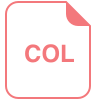
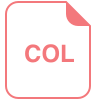