使用openssl对字符串进行aes加密的c语言程序,填充方式为PKCS7
时间: 2024-11-27 22:14:33 浏览: 33
在C语言中,使用OpenSSL库进行AES加密,特别是使用PKCS7填充方式,可以按照以下步骤编写程序:
首先,确保已经包含了OpenSSL相关的头文件,并链接了OpenSSL库。这是一个基本的例子,展示了如何使用AES-128-CBC模式以及PKCS7填充:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
#include <openssl/evp.h>
#define AES_BLOCK_SIZE 16
#define PKCS7_PADDING 1
void aes_encrypt_pkcs7(const char *plaintext, char *ciphertext, int keylen)
{
AES_KEY aes_key;
unsigned char iv[AES_BLOCK_SIZE]; // 初始化向量 (IV),通常随机生成
AES_set_encrypt_key((unsigned char*)keylen, keylen, &aes_key);
// 如果需要,这里可以设置随机的初始化向量
memset(iv, 0, sizeof(iv));
// 实际应用中可能需要从其他安全源获取IV
// 对明文进行PKCS7填充,直到达到AES块大小
int padding = AES_BLOCK_SIZE - (strlen(plaintext) % AES_BLOCK_SIZE); // 计算剩余字节数
for (int i = 0; i < padding; ++i) {
plaintext[strlen(plaintext)] = (char)(padding + i);
}
plaintext[strlen(plaintext)] = '\0'; // 添加终止符
// 加密
int len = strlen(plaintext);
int ciphertext_len = AES_BLOCK_SIZE * ((len + AES_BLOCK_SIZE - 1) / AES_BLOCK_SIZE);
unsigned char *cipher_text = malloc(ciphertext_len);
if (!cipher_text) {
printf("Memory allocation failed.\n");
return;
}
EVP_CipherInit_ex(&ctx, EVP_aes_128_cbc(), NULL, &aes_key, iv, 1); // 初始化加密上下文
EVP_CipherUpdate(&ctx, cipher_text, &ciphertext_len, (unsigned char *)plaintext, len);
EVP_CipherFinal_ex(&ctx, cipher_text + ciphertext_len, &padding);
// 结果可能包含填充,所以移除填充部分
for (int i = 0; i < padding; ++i) {
cipher_text[ciphertext_len - 1] -= i;
}
ciphertext = cipher_text;
printf("Encrypted string: %s\n", ciphertext);
}
int main()
{
const char *plaintext = "Hello, World!";
const size_t keylen = 16; // AES-128 需要16字节的密钥
aes_encrypt_pkcs7(plaintext, ciphertext, keylen);
free(ciphertext);
return 0;
}
```
注意:这个示例只用于演示目的,实际生产环境中,你需要确保正确的密钥管理和安全存储。
阅读全文
相关推荐
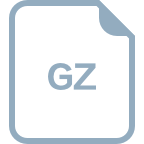

















