基于蚁群算法的带有障碍物的TSP旅行商问题matlab代码
时间: 2023-11-27 07:05:08 浏览: 71
Sure, here's an example of a MATLAB code for solving the TSP (Traveling Salesman Problem) with obstacles using the Ant Colony Optimization (ACO) algorithm:
```matlab
% TSP with obstacles using Ant Colony Optimization algorithm
% Assume the coordinates of cities are stored in the 'cities' matrix
% Assume the coordinates of obstacles are stored in the 'obstacles' matrix
% 'nCities' represents the number of cities; 'nAnts' represents the number of ants
nIterations = 100; % Number of iterations
nAnts = 20; % Number of ants
nCities = size(cities, 1); % Number of cities
alpha = 1; % Parameter controlling the influence of pheromone
beta = 5; % Parameter controlling the influence of distance
rho = 0.5; % Evaporation rate
Q = 100; % Pheromone deposit factor
% Calculate the distances between cities and obstacles
distances = zeros(nCities, nCities);
for i = 1:nCities
for j = 1:nCities
distances(i, j) = norm(cities(i, :) - cities(j, :));
end
end
% Initialize pheromone levels on edges and visibility matrix
pheromones = ones(nCities, nCities);
visibility = 1 ./ distances;
% Initialize best path and its length
bestPath = [];
bestPathLength = inf;
for iteration = 1:nIterations
% Initialize ant paths and visited cities
paths = zeros(nAnts, nCities);
visited = zeros(nAnts, nCities);
for ant = 1:nAnts
currentCity = randi(nCities); % Choose a random starting city
for step = 1:nCities-1
visited(ant, currentCity) = 1;
availableCities = find(visited(ant, :) == 0); % Get unvisited cities
% Calculate the probabilities for selecting the next city
probabilities = zeros(1, length(availableCities));
for i = 1:length(availableCities)
city = availableCities(i);
probabilities(i) = (pheromones(currentCity, city) ^ alpha) * (visibility(currentCity, city) ^ beta);
end
sumProbabilities = sum(probabilities);
probabilities = probabilities / sumProbabilities;
% Roulette wheel selection
rouletteWheel = cumsum(probabilities);
randomValue = rand();
nextCityIndex = find(rouletteWheel >= randomValue, 1);
nextCity = availableCities(nextCityIndex);
paths(ant, step) = nextCity;
currentCity = nextCity;
end
% Complete the path by returning to the starting city
remainingCities = find(visited(ant, :) == 0);
paths(ant, end) = remainingCities(1);
% Evaluate the path length
pathLength = 0;
for i = 1:nCities-1
pathLength = pathLength + distances(paths(ant, i), paths(ant, i+1));
end
% Update the best path if a shorter path is found
if pathLength < bestPathLength
bestPathLength = pathLength;
bestPath = paths(ant, :);
end
end
% Update pheromone levels
pheromones = (1 - rho) * pheromones; % Evaporation
for ant = 1:nAnts
for i = 1:nCities-1
pheromones(paths(ant, i), paths(ant, i+1)) = pheromones(paths(ant, i), paths(ant, i+1)) + Q / bestPathLength;
end
end
end
% Plot the best path
figure;
plot(cities(:, 1), cities(:, 2), 'ro'); % Plot cities as red circles
hold on;
plot(cities(bestPath, 1), cities(bestPath, 2), 'b-'); % Plot best path as blue line
plot(obstacles(:, 1), obstacles(:, 2), 'kx'); % Plot obstacles as black crosses
title('TSP with Obstacles');
xlabel('X');
ylabel('Y');
legend('Cities', 'Best Path', 'Obstacles');
```
Please note that you would need to provide the coordinates of cities and obstacles in their respective matrices (`cities` and `obstacles`). Additionally, you can adjust the parameters (`nIterations`, `nAnts`, `alpha`, `beta`, `rho`, `Q`) based on your requirements.
I hope this helps! Let me know if you have any further questions.
相关推荐
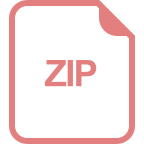














