通过POSIX线程(pthread)库,用C语言写一个简单的求定积分的并行编程的代码例子
时间: 2024-09-23 16:11:49 浏览: 29
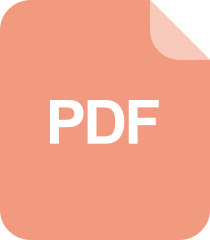
C语言多线程编程:使用POSIX线程(pthread)
在C语言中,我们可以使用POSIX线程库(pthread)来实现简单的问题求解的并行计算,例如求定积分。下面是一个简单的示例,我们将使用两个线程分别处理积分的上下限部分:
```c
#include <stdio.h>
#include <math.h>
#include <pthread.h>
#define PI 3.14159
// 定义积分函数
double integral(double a, double b) {
return (b - a) * sin(a);
}
// 线程执行函数,计算定积分的一部分
void* integrate_part(void* arg) {
double* range = (double*)arg;
double result = integral(*range, (*range) + PI / 4);
printf("Thread %d: Integral from %.2f to %.2f is %.6f\n", pthread_self(), *range, *range + PI / 4, result);
return NULL;
}
int main() {
double lower_limit = 0.0;
double upper_limit = PI;
// 创建两个线程
pthread_t thread1, thread2;
if (pthread_create(&thread1, NULL, integrate_part, &lower_limit) != 0) {
perror("Creating first thread");
exit(1);
}
if (pthread_create(&thread2, NULL, integrate_part, &upper_limit) != 0) {
perror("Creating second thread");
exit(1);
}
// 等待线程完成
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Total integral from 0 to %.2f Pi is %.6f\n", PI, integral(lower_limit, upper_limit));
return 0;
}
```
在这个例子中,我们创建了两个线程,每个线程负责计算定积分的一半范围。主线程创建了这两个线程,并等待它们完成。最后,主线程将两个部分的结果合并,得到整个定积分。
阅读全文
相关推荐
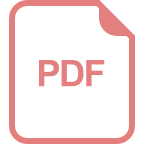
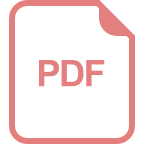




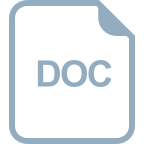
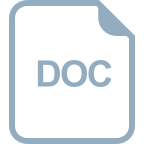

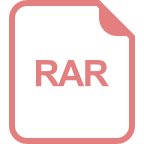
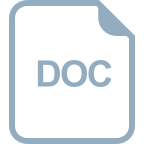
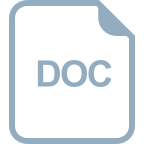
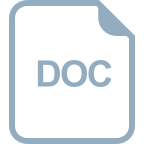
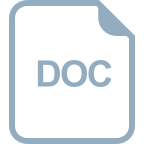
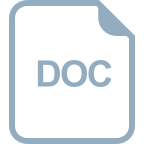
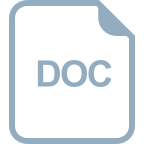
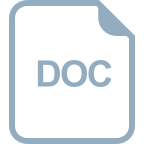
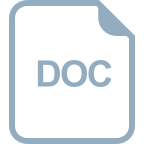