如何用C语言编写统计字符串中字符的出现次数?
时间: 2024-11-22 20:44:15 浏览: 169
在C语言中,可以使用循环结构和数组来统计字符串中每个字符的出现次数。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
void count_chars(char *str) {
int char_count[256] = {0}; // 创建一个大小为256的数组,用于存储ASCII字符的计数,因为ASCII码有256个可能值
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') { // 对于小写字母
char_count[str[i] - 'a']++;
} else if (str[i] >= 'A' && str[i] <= 'Z') { // 对于大写字母
char_count[str[i] - 'A']++;
} else if (str[i] >= '0' && str[i] <= '9') { // 对于数字
char_count[str[i] - '0']++;
} else { // 其他字符如空格、标点等
char_count[str[i]]++; // 直接累加其他非字母数字字符
}
}
// 输出每个字符及其出现次数
for (int i = 0; i < 256; i++) {
if (char_count[i] > 0) {
printf("'%c': %d times\n", i + 'a', char_count[i]); // 输出小写字母
printf("'%c': %d times\n", i + 'A', char_count[i]); // 输出大写字母
}
}
}
int main() {
char str[] = "Hello, World! 123";
count_chars(str);
return 0;
}
```
在这个程序中,`count_chars` 函数遍历输入的字符串,根据字符的ASCII值更新数组 `char_count` 的相应元素。然后在主函数中调用这个函数,并打印出每个字符及其出现次数。
阅读全文
相关推荐
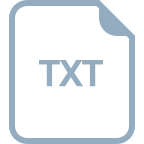
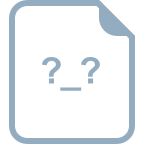
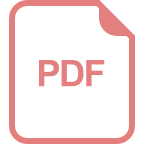


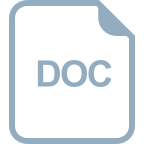
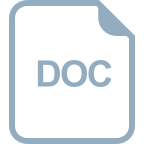
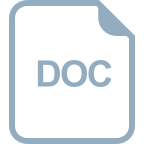










