springboot中文件上传下载
时间: 2024-01-21 12:14:54 浏览: 110
以下是SpringBoot中文件上传和下载的步骤:
1. 创建SpringBoot项目并导入相关依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
```
2. 在application.properties文件中配置文件保存路径:
```properties
filePath=E:/springboot_save_file/
```
3. 创建文件上传的Controller:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import java.io.File;
import java.io.IOException;
@Controller
public class FileUploadController {
@Value("${filePath}")
private String filePath;
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload");
return "redirect:/";
}
try {
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
File destFile = new File(filePath + fileName);
file.transferTo(destFile);
redirectAttributes.addFlashAttribute("message", "File uploaded successfully");
} catch (IOException e) {
e.printStackTrace();
redirectAttributes.addFlashAttribute("message", "Failed to upload file");
}
return "redirect:/";
}
}
```
4. 创建文件下载的Controller:
```java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
@Controller
public class FileDownloadController {
@Value("${filePath}")
private String filePath;
@GetMapping("/download/{fileName:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName) {
Path file = Paths.get(filePath + fileName);
Resource resource;
try {
resource = new UrlResource(file.toUri());
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
}
```
5. 创建Thymeleaf模板文件index.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring Boot File Upload/Download</title>
</head>
<body>
<h1>File Upload/Download</h1>
<form method="post" action="/upload" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="submit" value="Upload" />
</form>
<p th:text="${message}"></p>
<hr/>
<h2>Download Files:</h2>
<ul>
<li th:each="file : ${files}">
<a th:href="@{/download/{fileName}(fileName=${file})}" th:text="${file}"></a>
</li>
</ul>
</body>
</html>
```
6. 运行SpringBoot应用,并访问http://localhost:8080/,即可进行文件上传和下载操作。
阅读全文
相关推荐
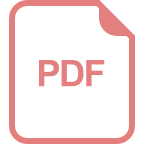



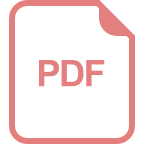
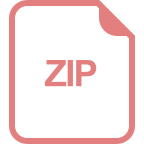
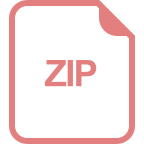
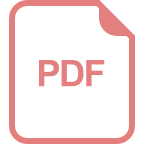
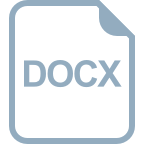
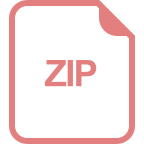
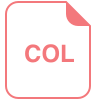
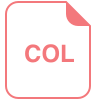
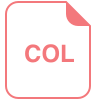
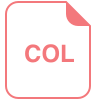
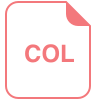
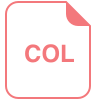