C语言中如何在输入函数输入参数后再确定数组大小
时间: 2024-09-13 08:13:43 浏览: 27
在C语言中,如果要在读取用户输入之后动态确定数组大小,通常会在输入阶段先询问用户需要多少个元素,然后将这个数量传递给创建数组的函数。这里有一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个函数接收用户输入并分配相应大小的数组
int* create_array(int count) {
int* arr = (int*)malloc(count * sizeof(int)); // 分配内存
if (arr != NULL) {
printf("Please enter %d numbers:\n", count);
for (int i = 0; i < count; i++) {
scanf("%d", &arr[i]); // 读取每个元素
}
return arr;
} else {
printf("Memory allocation failed.\n");
exit(1);
}
}
int main() {
int num_elements;
printf("How many elements do you want to store? ");
scanf("%d", &num_elements); // 获取用户输入
// 确保分配足够的内存
if (num_elements > 0) {
int* arr = create_array(num_elements);
// ... 使用数组
free(arr); // 释放内存
} else {
printf("Invalid input.\n");
}
return 0;
}
```
在这个例子中,`create_array()`函数会根据`count`参数来创建数组,并提示用户输入指定数量的值。但在实际使用时,记得处理可能出现的问题,如检查输入是否合法、内存分配是否成功等。
相关推荐
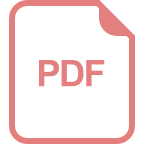
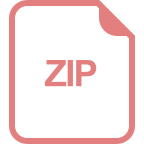
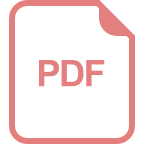














