删除单链表中最大值节点并返回值,包括增删改查,用python实现
时间: 2024-10-27 10:13:28 浏览: 13
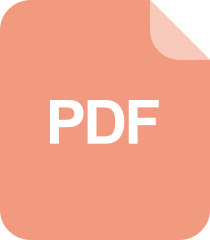
Python实现的一个简单LRU cache
在Python中,我们可以创建一个单链表节点类,并实现删除最大值节点的功能。这里先假设我们有一个`ListNode`类,表示链表节点,包含`val`属性存储数据和`next`属性指向下一个节点。以下是删除最大值节点的步骤:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def find_max_and_remove(head):
# 如果链表为空或只有一个元素,直接返回
if not head or not head.next:
return None if not head else head.val
# 定义两个指针,一个跟踪当前的最大值,一个遍历链表
max_val = head.val
current = head
while current:
if current.val > max_val:
max_val = current.val
current = current.next
# 更新最大值节点为None,然后找到它并替换
for node in head:
if node.val == max_val:
node.val = None
break
# 返回最大值
return max_val
# 示例用法
# 创建一个链表 [3, 2, 4, 5, 6]
head = ListNode(3)
head.next = ListNode(2)
head.next.next = ListNode(4)
head.next.next.next = ListNode(5)
head.next.next.next.next = ListNode(6)
max_value = find_max_and_remove(head)
print(f"删除后的链表: ", [node.val for node in head if node is not None])
阅读全文
相关推荐
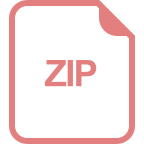
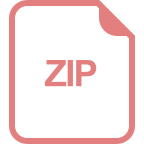






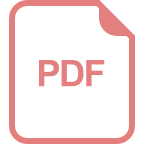
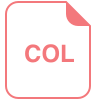
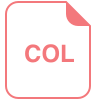
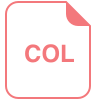
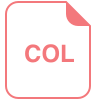
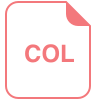
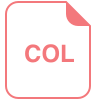


