fastapi中的item
时间: 2024-04-24 19:20:38 浏览: 80
在FastAPI中,"item"是一个通用的术语,用于表示一个特定的数据项或资源。它可以是一个数据库中的记录、一个文件、一个用户、一篇文章等等。在FastAPI中,我们可以使用"item"来表示我们要处理的特定数据。
通常情况下,我们使用FastAPI来创建RESTful API,通过定义路由和处理函数来处理不同的HTTP请求。当我们需要对某个特定的数据项进行操作时,可以使用"item"来表示这个数据项。
例如,如果我们正在构建一个博客应用程序,我们可以定义一个路由来处理获取特定文章的请求:
```python
from fastapi import FastAPI
app = FastAPI()
@app.get("/articles/{article_id}")
async def get_article(article_id: int):
# 通过article_id获取特定文章的逻辑
# ...
return {"article_id": article_id, "title": "Example Article"}
```
在上面的例子中,"item"就是指代文章(article),通过`/articles/{article_id}`路由获取特定文章的信息。
相关问题
fastapi 中 使用 queue
在 FastAPI 中使用队列可以使用 Python 内置的 `queue` 模块,或者第三方的异步队列库 `aiorq`。下面是两个示例:
使用 Python 内置的 `queue` 模块:
```python
import asyncio
from fastapi import FastAPI
from queue import Queue
app = FastAPI()
q = Queue()
async def worker():
while True:
item = await asyncio.get_event_loop().run_in_executor(None, q.get)
# 处理队列中的任务,这里只是简单地打印出来
print(f"Processing {item}")
q.task_done()
@app.post("/task")
async def add_task(item: str):
q.put(item)
return {"message": "Task added to queue"}
if __name__ == "__main__":
asyncio.create_task(worker())
uvicorn.run("module_name:app", host="0.0.0.0", port=8000, log_level="info")
```
在上面的示例中,`worker` 协程不断地从队列中获取任务,并进行处理。`add_task` 函数将任务添加到队列中。在 Uvicorn 的 `run` 函数中,将 `module_name` 替换为你的应用程序模块名,例如 `myapp.main`。
使用 `aiorq` 库:
```python
import asyncio
from fastapi import FastAPI
from aiorq import Queue
app = FastAPI()
q = Queue()
async def worker():
while True:
item = await q.get()
# 处理队列中的任务,这里只是简单地打印出来
print(f"Processing {item}")
await q.task_done()
@app.post("/task")
async def add_task(item: str):
await q.put(item)
return {"message": "Task added to queue"}
if __name__ == "__main__":
asyncio.create_task(worker())
uvicorn.run("module_name:app", host="0.0.0.0", port=8000, log_level="info")
```
在上面的示例中,`worker` 协程不断地从队列中获取任务,并进行处理。`add_task` 函数将任务添加到队列中。请确保在安装并导入 `aiorq` 库。在 Uvicorn 的 `run` 函数中,将 `module_name` 替换为你的应用程序模块名,例如 `myapp.main`。
fastapi 中使用redis
FastAPI 是一个轻量级的 Web 框架,而 Redis 是一个流行的内存数据存储系统,常用于缓存、会话管理和实时数据存储。在 FastAPI 中集成 Redis,可以帮助提高应用性能和扩展性。以下是如何在 FastAPI 中使用 Redis 的简单步骤:
1. 安装依赖:首先,你需要安装 `aioredis` 库,它是异步操作 Redis 的 Python 接口。可以通过 pip 安装:
```
pip install aioredis
```
2. 配置连接:在你的应用中,设置一个连接到 Redis 的异步客户端。你可以创建一个单例的 Redis 连接实例,以便在整个应用中复用:
```python
import aioredis
redis_pool = None
async def get_redis_connection():
global redis_pool
if not redis_pool:
redis_pool = await aioredis.create_pool('redis://localhost:6379')
return await redis_pool.get_client()
# 在 FastAPI 的服务启动时(或配置文件中),初始化连接
app = FastAPI()
app.state.redis = get_redis_connection()
```
3. 使用 Redis:在你的 FastAPI 函数中,你可以使用连接来存储和检索数据。例如,存储数据:
```python
async def set_item(item_key, item_value):
redis = app.state.redis
await redis.set(item_key, item_value)
```
获取数据:
```python
async def get_item(item_key):
redis = app.state.redis
return await redis.get(item_key)
```
4. 键值对操作:你可以使用 Redis 提供的多种操作,如哈希、集合、有序集合等,具体取决于你的应用需求。
阅读全文
相关推荐
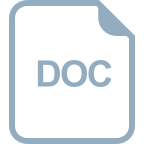
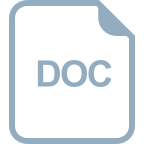
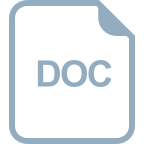
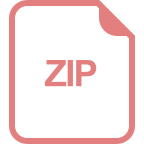
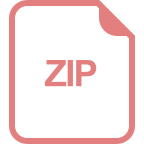
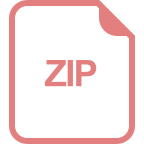
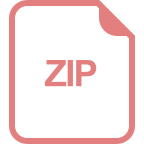
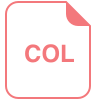
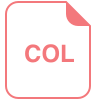
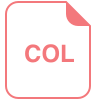
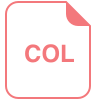
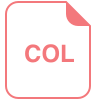




