判断TCHAR*为空
时间: 2023-09-17 18:09:01 浏览: 235
要判断TCHAR*是否为空,可以使用以下方法:
```cpp
TCHAR* str = ...; // 你的TCHAR*变量
if (str == NULL) {
// str为空
// 进行处理...
} else {
// str不为空
// 进行处理...
}
```
或者使用以下方法:
```cpp
TCHAR* str = ...; // 你的TCHAR*变量
if (str[0] == '\0') {
// str为空
// 进行处理...
} else {
// str不为空
// 进行处理...
}
```
这两种方法都可以判断TCHAR*变量是否为空。第一种方法是直接比较指针是否为NULL,第二种方法是判断字符串的第一个字符是否为'\0'。根据你的具体情况选择适合的方法。
相关问题
判断TCHAR字符串是否有值
可以使用_tcslen函数来判断TCHAR字符串是否有值,如果返回值大于0,则说明该字符串有值,否则为空字符串。
以下是一个示例代码:
```c++
#include <tchar.h>
#include <iostream>
int _tmain(int argc, _TCHAR* argv[])
{
TCHAR str[] = _T("Hello World");
if (_tcslen(str) > 0)
{
std::wcout << _T("The string is not empty.") << std::endl;
}
else
{
std::wcout << _T("The string is empty.") << std::endl;
}
return 0;
}
```
用TCHAR argv接收的参数转换为int型
可以使用 _ttoi 函数将 TCHAR 类型的字符串参数转换为整型。
示例代码:
```c++
#include <tchar.h>
#include <iostream>
int _tmain(int argc, TCHAR* argv[])
{
if (argc < 2)
{
std::cout << "Please enter an integer parameter!" << std::endl;
return 0;
}
int param = _ttoi(argv[1]);
std::cout << "The parameter is: " << param << std::endl;
return 0;
}
```
在上述示例代码中,我们使用 _ttoi 函数将 TCHAR 类型的 argv[1] 参数转换为整型。需要注意的是,如果没有传入参数,则 argc 的值为 1,argv[0] 为程序名,argv[1] 为空,因此需要先判断参数数量是否正确。
阅读全文
相关推荐
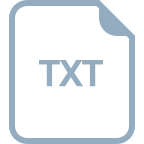




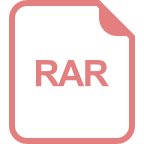
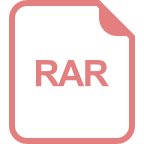
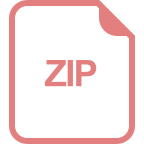
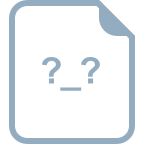
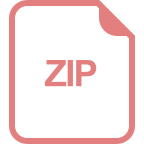
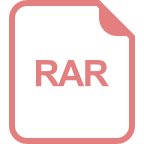
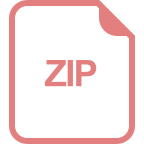