如何使用PyTorch在多台树莓派(Raspberry Pi)上实现模型数据的并行推理?能否提供相关的代码示例?
时间: 2024-10-22 17:20:39 浏览: 22
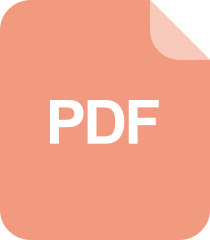
pytorch构建多模型实例
在多台树莓派(Raspberry Pi)上实现模型数据的并行推理通常通过分布式计算框架如Dask或Horovod等工具来协同工作。对于PyTorch,我们可以借助torch.distributed库,它支持在多GPU或多节点环境中进行模型训练和推理。
以下是一个简化的步骤和代码示例:
1. 安装必要的库:
```
pip install torch torchvision dask[distributed] horovod[tensorflow]
```
2. 初始化分布式环境:
```python
import torch.distributed as dist
from dask.distributed import Client
# 如果有多台树莓派,需要设置适当的网络通信方式(如gRPC、TCP)
if __name__ == "__main__":
client = Client('scheduler_address:port') # 替换为实际的调度器地址和端口
print(f"Connected to {client}")
dist.init_process_group(backend='nccl', init_method='tcp://localhost:5000')
```
3. 加载模型并在每个设备上分布:
```python
model = YourModel() # 按需替换为您的模型
model = nn.parallel.DistributedDataParallel(model, device_ids=[dist.get_rank()])
```
4. 推理数据:
```python
with torch.no_grad():
input_data = ... # 获取待预测的数据
output = model(input_data)
```
5. 数据处理和结果收集:
```python
if dist.get_rank() == 0:
# 仅主节点接收所有结果并聚合
outputs = [output for _ in range(dist.get_world_size())]
results = torch.cat(outputs, dim=0) # 或其他适合您任务的聚合方式
# 对结果进行进一步操作...
```
注意:这只是一个基础示例,实际应用中需要处理网络同步、错误处理以及更复杂的资源分配。同时,由于树莓派性能有限,对于大规模的深度学习任务,可能更适合使用更强大且高效的服务器集群。
阅读全文
相关推荐
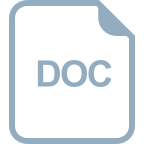
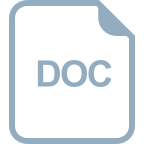

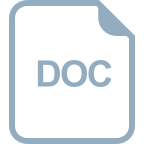
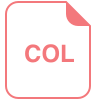
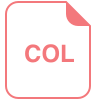
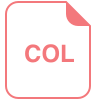
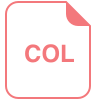
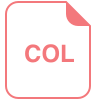
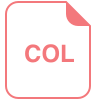
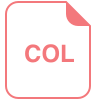
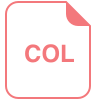
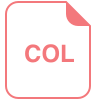
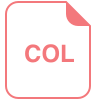
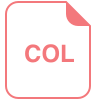
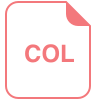
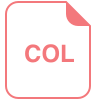
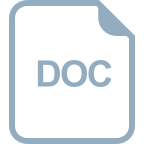