YOLO与神经网络的部署策略:高效部署模型,赋能实际场景
发布时间: 2024-08-17 19:12:45 阅读量: 19 订阅数: 21 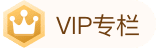
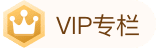
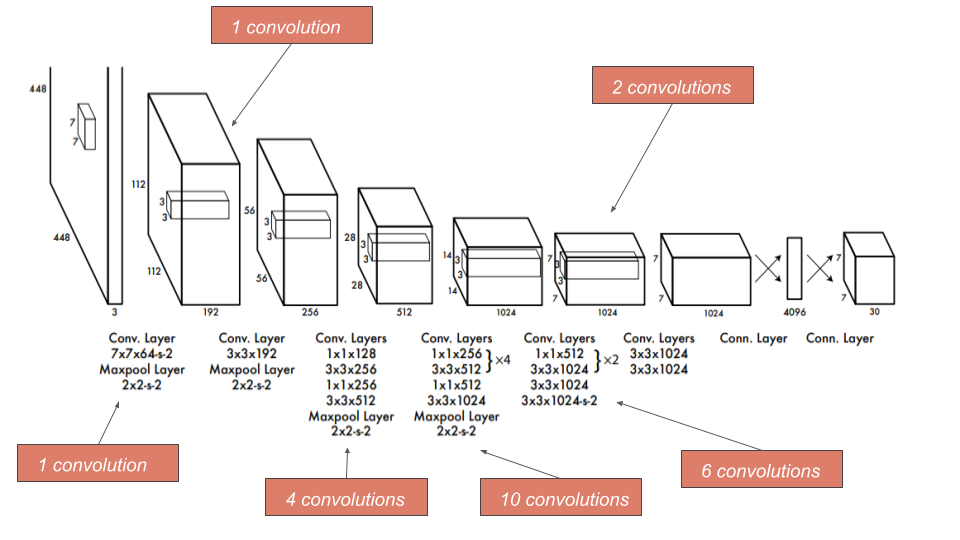
# 1. YOLO目标检测算法简介
YOLO(You Only Look Once)是一种单阶段目标检测算法,它将目标检测任务转化为回归问题,一次性预测目标的类别和边界框。与传统的两阶段算法(如Faster R-CNN)相比,YOLO具有更快的速度和更高的准确率。
YOLO算法的核心思想是将输入图像划分为一个网格,并为每个网格单元预测一个边界框和一组类别概率。每个网格单元只负责预测与其重叠程度最大的目标,从而减少了计算量。此外,YOLO使用了一个单一的卷积神经网络(CNN)来完成整个检测过程,避免了多阶段算法中繁琐的候选区域生成和特征提取步骤。
# 2. 神经网络模型部署基础
神经网络模型的部署是将训练好的模型应用于实际场景的过程。在部署过程中,需要考虑模型的优化、压缩、部署平台选择等因素。
### 2.1 模型优化与压缩
模型优化与压缩旨在减小模型的大小和计算复杂度,同时保持或提高模型的精度。常见的优化和压缩技术包括:
#### 2.1.1 模型剪枝与量化
**模型剪枝**:通过移除不重要的神经元和连接来减小模型的大小。
```python
import tensorflow as tf
# 创建一个模型
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 剪枝模型
pruned_model = tf.keras.models.prune_low_magnitude(model, 0.5)
```
**量化**:将浮点权重和激活转换为低精度格式,如int8或int16。
```python
import tensorflow as tf
# 创建一个模型
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 量化模型
quantized_model = tf.keras.models.quantize_model(model)
```
#### 2.1.2 知识蒸馏与迁移学习
**知识蒸馏**:将教师模型的知识转移到较小的学生模型中,以提高学生的精度。
```python
import tensorflow as tf
# 创建教师模型和学生模型
teacher_model = tf.keras.models.Sequential([
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
student_model = tf.keras.models.Sequential([
tf.keras.layers.Dense(5, activation='relu'),
tf.keras.layers.Dense(5, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 知识蒸馏
student_model.compile(
optimizer='adam',
loss=tf.keras.losses.MeanSquaredError(),
metrics=['accuracy']
)
student_model.fit(
teacher_model.predict(X_train),
y_train,
epochs=10
)
```
**迁移学习**:使用在不同数据集上训练的预训练模型作为基础,并对其进行微调以适应新任务。
```python
import tensorflow as tf
# 创建一个预训练模型
pre_trained_model = tf.keras.applications.VGG16(
include_top=False,
weights='imagenet'
)
# 创建一个新模型
new_model = tf.keras.models.Sequential([
pre_trained_model,
tf.keras.layers.Dense(10,
```
0
0
相关推荐
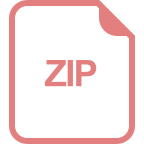
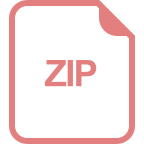
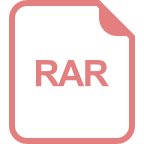
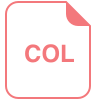
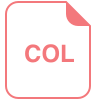
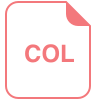
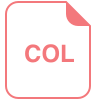
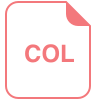
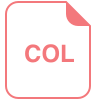