编写matlab.h和matlab.c
时间: 2024-12-18 07:13:29 浏览: 8
编写`matlab.h`和`matlab.c`的过程如下:
**matlab.h**(头文件,定义函数原型)
```c
#ifndef MATLAB_H
#define MATLAB_H
#include <stdlib.h> // 包含基本内存管理函数如malloc, free
#include <stdbool.h> // 包含布尔类型
// 矩阵结构定义
typedef struct {
int row, col;
float *dat;
} Matrix;
// 创建矩阵函数原型
Matrix* CreateMatrix(int row, int col);
Matrix* CreateMatrixIdentity(int row, int col);
// 销毁矩阵函数原型
void DestroyMatrix(Matrix **mat);
// 访问矩阵元素函数原型
float* MatrixAt(int row, int col, const Matrix *mat);
// 获取内存使用量函数原型
size_t GetTotalMemoryUsedByMatrix();
#endif // MATLAB_H
```
**matlab.c**(源文件,实现函数)
```c
#include "matlab.h" // 引入本头文件
// 实现函数
Matrix* CreateMatrix(int row, int col) {
// 检查输入合法性
if (row <= 0 || col <= 0) {
return NULL;
}
// 动态分配内存
Matrix *newMat = (Matrix*) malloc(sizeof(Matrix));
newMat->row = row;
newMat->col = col;
newMat->dat = (float*) malloc(row * col * sizeof(float));
for (int i = 0; i < row * col; ++i) {
newMat->dat[i] = 0.0f; // 初始化为0
}
return newMat;
}
Matrix* CreateMatrixIdentity(int row, int col) {
if (row != col || row <= 0) {
return NULL;
}
Matrix *newMat = CreateMatrix(row, col);
if (!newMat) {
return NULL;
}
for (int i = 0; i < row; ++i) {
newMat->dat[i * col + i] = 1.0f; // 对角线元素置1
}
return newMat;
}
void DestroyMatrix(Matrix **mat) {
if (*mat && (*mat)->dat) {
free(*mat->dat); // 释放dat区域的内存
free(*mat); // 释放整个矩阵结构
*mat = NULL; // 更新指针为NULL
}
}
float* MatrixAt(int row, int col, const Matrix *mat) {
if (row >= 0 && row < mat->row && col >= 0 && col < mat->col) {
return &mat->dat[row * mat->col + col]; // 返回元素地址
} else {
return NULL; // 如果越界,返回NULL
}
}
size_t GetTotalMemoryUsedByMatrix() {
size_t totalMemory = 0;
if (matlab_global_list) { // 假设有一个全局列表存放所有活跃矩阵
for (Matrix *mat : matlab_global_list) {
if (mat && mat->dat) {
totalMemory += mat->row * mat->col * sizeof(float);
}
}
}
return totalMemory;
}
```
在这个例子中,我们假设存在一个全局列表`matlab_global_list`用于跟踪活跃的矩阵,以便在`GetTotalMemoryUsedByMatrix`函数中计算总内存。实际项目中,可以根据需求调整内存管理和跟踪策略。务必记得在main.c中初始化并维护这个列表,并确保每个矩阵在适当的时候添加到或移除出这个列表。
阅读全文
相关推荐













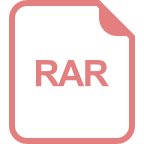



