C语言写建立一个双链表,包含插入元素、删除元素、查找元素、显示所有元素等基本操作的代码
时间: 2024-06-12 21:09:33 浏览: 10
双链表的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 双链表的结构体
struct Node {
int data;
struct Node* prev;
struct Node* next;
};
// 在链表末尾插入元素
void append(struct Node** head_ref, int new_data) {
// 如果链表为空,则新元素为头结点
if (*head_ref == NULL) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->prev = NULL;
new_node->next = NULL;
*head_ref = new_node;
return;
}
// 定义一个指针指向链表最后一个元素
struct Node* last = *head_ref;
while (last->next != NULL) {
last = last->next;
}
// 在链表末尾插入新元素
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->prev = last;
new_node->next = NULL;
last->next = new_node;
return;
}
// 在链表中删除元素
void deleteNode(struct Node** head_ref, int key) {
// 如果链表为空,则返回
if (*head_ref == NULL) {
return;
}
// 定义一个指针指向要删除的元素
struct Node* del = *head_ref;
while (del != NULL && del->data != key) {
del = del->next;
}
// 如果找不到要删除的元素,则返回
if (del == NULL) {
return;
}
// 如果要删除的元素是头结点,则改变头结点
if (*head_ref == del) {
*head_ref = del->next;
}
// 如果要删除的元素有前一个元素,则改变前一个元素的next指针
if (del->prev != NULL) {
del->prev->next = del->next;
}
// 如果要删除的元素有后一个元素,则改变后一个元素的prev指针
if (del->next != NULL) {
del->next->prev = del->prev;
}
// 释放要删除的元素的内存
free(del);
return;
}
// 在链表中查找元素
struct Node* search(struct Node* head, int key) {
// 定义一个指针指向要查找的元素
struct Node* curr = head;
while (curr != NULL && curr->data != key) {
curr = curr->next;
}
// 返回要查找的元素
return curr;
}
// 显示所有元素
void display(struct Node* node) {
// 定义一个指针遍历链表
struct Node* last = NULL;
printf("Forward traversal: ");
while (node != NULL) {
printf("%d ", node->data);
last = node;
node = node->next;
}
printf("\nBackward traversal: ");
while (last != NULL) {
printf("%d ", last->data);
last = last->prev;
}
}
// 主函数
int main() {
struct Node* head = NULL; // 定义一个指针指向链表的头结点
append(&head, 6); // 在链表末尾插入元素6
append(&head, 8); // 在链表末尾插入元素8
append(&head, 2); // 在链表末尾插入元素2
append(&head, 5); // 在链表末尾插入元素5
display(head); // 显示所有元素
deleteNode(&head, 2); // 删除元素2
display(head); // 显示所有元素
struct Node* search_result = search(head, 8); // 在链表中查找元素8
printf("Search result: %d\n", search_result->data); // 显示查找结果
return 0;
}
```
相关推荐
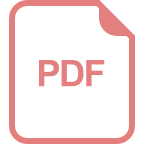
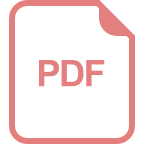
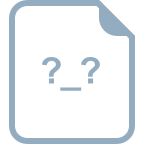














