从0开始写代码,使用bilstm-crf识别实体,有一个维护数据集(包含机械名称,故障点位,故障描述,维修方法,使用BIO模式标注)。如何写代码。请详细说出思路
时间: 2024-10-04 09:02:22 浏览: 35
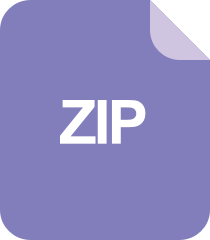
基于Pytorch的BERT-IDCNN-BILSTM-CRF中文实体识别实现
在Python中使用`Keras`库结合`TensorFlow`的`Bidirectional LSTM`(双向长短期记忆网络)和`Conditional Random Field`(CRF)来实现命名实体识别(NER),针对您提到的维护数据集,可以按照以下步骤编写代码:
1. **数据预处理**:
- 导入必要的库如`pandas`, `numpy`, `nltk`等。
- 加载并清洗数据,将文本分割成单词(tokenization),同时对标签进行编码(例如,使用`BIO`标记体系)。
```python
import pandas as pd
from sklearn.model_selection import train_test_split
data = pd.read_csv('maintenance_dataset.csv')
X = data['texts']
y = data['labels']
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
```
2. **词汇和标签构建**:
- 创建词典和标签索引,用于序列化输入和输出。
```python
from keras.preprocessing.text import Tokenizer
from keras.utils import to_categorical
tokenizer = Tokenizer()
tokenizer.fit_on_texts(X_train)
vocab_size = len(tokenizer.word_index) + 1
max_length = max([len(text.split()) for text in X_train])
label_encoder = LabelEncoder()
y_train_encoded = label_encoder.fit_transform(y_train)
y_train_categorical = to_categorical(y_train_encoded)
```
3. **特征提取**:
- 将文本转换为数字序列,通常会包括填充和截断。
```python
X_train_sequences = tokenizer.texts_to_sequences(X_train)
X_test_sequences = tokenizer.texts_to_sequences(X_test)
# Padding or truncating sequences to the same length
X_train_padded = pad_sequences(X_train_sequences, maxlen=max_length, padding='post', truncating='post')
X_test_padded = pad_sequences(X_test_sequences, maxlen=max_length, padding='post', truncating='post')
```
4. **构建LSTM-CRF模型**:
- 使用`keras_contrib.layers`中的`TimeDistributed`和`CRF`层。
```python
from keras_contrib.layers import CRF
input_shape = (max_length, vocab_size)
lstm_units = 64
model = Sequential([
Embedding(vocab_size, lstm_units, input_length=max_length),
Bidirectional(LSTM(lstm_units)),
TimeDistributed(Dense(lstm_units, activation='relu')),
CRF(num_tags=label_encoder.classes_)
])
```
5. **模型编译和训练**:
- 设置损失函数、优化器和评估指标。
```python
model.compile(optimizer='adam', loss=crf_loss_function, metrics=['accuracy'])
history = model.fit(X_train_padded, y_train_categorical, epochs=10, validation_data=(X_test_padded, y_test_categorical), batch_size=32)
```
6. **预测和评估**:
- 对测试集应用模型,并查看性能。
```python
y_pred = model.predict(X_test_padded)
y_pred_decode = decode_predictions(y_pred, label_encoder, max_length)
```
阅读全文
相关推荐
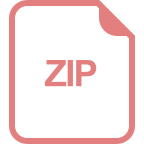
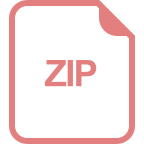
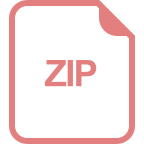




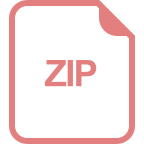
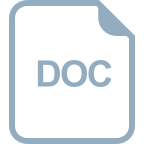
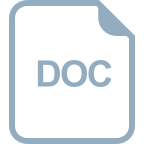
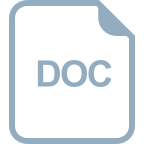






