cpp 将数字接受为字符串类型
时间: 2024-10-24 17:16:08 浏览: 9
在C++中,如果你有一个数字以字符串形式存在,你可以使用`atoi()` 或者 `stoi()` 函数将其转换为整数。这里是两个函数的基本用法:
1. 使用 `atoi()`[^2]:
```cpp
const char strNum[] = "123"; // 假设你有一个字符串 "123"
int numAsInt = atoi(strNum); // 这将会尝试将字符串转换为整数
```
2. 使用 `stoi()`[^1], 它更现代且提供了更好的错误处理:
```cpp
std::string strNum = "123"; // 注意这里我们使用std::string而非字符数组
try {
int numAsInt = std::stoi(strNum);
} catch (const std::invalid_argument& e) {
// 处理转换失败的情况
}
```
`atoi()` 对于简单的字符串转换有效,但如果字符串不是有效的整数表示(如包含非数字字符),则可能会导致未定义的行为。而 `stoi()` 可以捕获这种异常,使得程序更加健壮。
相关问题
输入一个字符串,统计英文字母 空格 数字 和其他字符的字数 要求,定义函数完成,个数通过指针返回
在编程中,你可以定义一个函数来实现这个功能。通常我们会采用C++或Python这样的语言,这里我会给出一个简单的C++示例:
```cpp
#include <iostream>
using namespace std;
// 函数声明,接收一个字符串并返回三个整数指针,分别指向字母、空格和非字母数字字符的计数
void countCharacters(const string& str, int* alphaCount, int* spaceCount, int* otherCount);
int main() {
string input;
cout << "请输入一个字符串: ";
getline(cin, input); // 使用getline处理包含空格的输入
int alphaCount = 0, spaceCount = 0, otherCount = 0;
countCharacters(input, &alphaCount, &spaceCount, &otherCount);
cout << "字母数: " << *alphaCount << endl;
cout << "空格数: " << *spaceCount << endl;
cout << "其他字符数: " << *otherCount << endl;
return 0;
}
// 定义countCharacters函数
void countCharacters(const string& str, int* alphaCount, int* spaceCount, int* otherCount) {
for (char c : str) {
if (isalpha(c)) { // 判断是否为字母
(*alphaCount)++;
} else if (isspace(c)) { // 判断是否为空格
(*spaceCount)++;
} else { // 其他字符(不是字母也不是空格)
(*otherCount)++;
}
}
}
```
在这个例子中,我们首先定义了一个`countCharacters`函数,它接受一个字符串和三个整型指针作为参数。然后遍历字符串中的每个字符,使用`isalpha`和`isspace`函数判断字符类型,并更新相应的计数器。最后,在主函数中调用这个函数并通过指针获取结果。
从键盘输入C语言风格字符串abCD123□□@#&,利用函数统计其中英文字符、数字、空格和其他字符的个数。全部用c++完成
在C++中,你可以使用`std::string`类来处理字符串,并结合遍历和条件判断来统计字符种类。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <cctype> // 包含用于处理字符类型的库
void countCharacters(const std::string& str) {
int asciiCount = 0;
int digitCount = 0;
int spaceCount = 0;
int otherCount = 0;
for (char c : str) {
if (isalpha(c)) { // 检查是否为字母
if (isupper(c)) {
asciiCount++;
} else if (islower(c)) {
asciiCount++;
}
} else if (isdigit(c)) { // 检查是否为数字
digitCount++;
} else if (isspace(c)) { // 检查是否为空格
spaceCount++;
} else { // 其他字符
otherCount++;
}
}
std::cout << "英文字符: " << asciiCount << "\n";
std::cout << "数字: " << digitCount << "\n";
std::cout << "空格: " << spaceCount << "\n";
std::cout << "其他字符: " << otherCount << "\n";
}
int main() {
std::string input = "abCD123□□@#&";
countCharacters(input);
return 0;
}
```
在这个例子中,我们定义了一个名为`countCharacters`的函数,它接收一个`const std::string`作为参数。通过`for-each`循环遍历字符串中的每个字符,然后使用`std::isalpha`, `std::isdigit`, 和 `std::isspace` 函数检查字符的类型。最后,在`main`函数中,我们创建一个字符串并调用这个函数来统计。
阅读全文
相关推荐
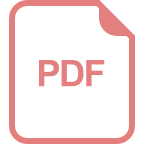
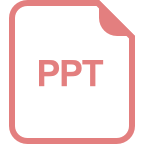
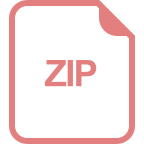
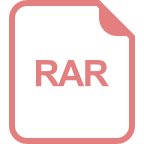
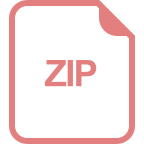
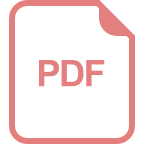
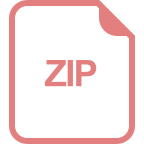
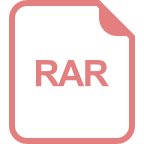
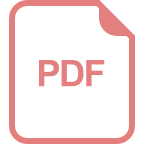
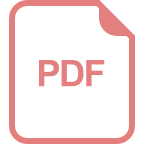
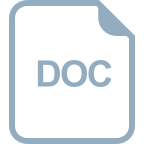
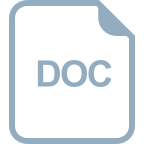
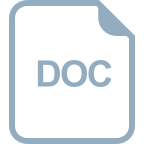
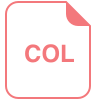
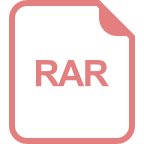
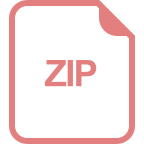