react获取dom
时间: 2023-09-03 21:07:56 浏览: 48
在 React 中,通常我们不直接操作 DOM,而是使用组件和虚拟 DOM 进行交互。然而,有时我们可能需要获取某个组件渲染后的真实 DOM 元素。在这种情况下,可以使用 `ref` 来获取 DOM。
首先,在组件的构造函数中创建一个 ref 对象:
```jsx
constructor(props) {
super(props);
this.myRef = React.createRef();
}
```
然后,在需要获取 DOM 的元素上添加 `ref` 属性,并将之前创建的 ref 对象赋值给它:
```jsx
render() {
return <div ref={this.myRef}>Hello, World!</div>;
}
```
现在,`this.myRef.current` 就是对应 DOM 元素的引用了。你可以在组件的生命周期方法或事件处理函数中使用它:
```jsx
componentDidMount() {
console.log(this.myRef.current); // 输出对应的 DOM 元素
}
```
请注意,`ref` 只能在类组件中使用。如果你正在使用函数组件,可以使用 `useRef` 这个 Hook 来达到同样的效果。
相关问题
react获取dom节点
在 React 中获取 DOM 节点有多种方法,以下是其中的一些:
1. 使用 ref 属性
可以在组件中通过 ref 属性获取 DOM 节点。例如,可以在 render 方法中创建一个 div 元素,并将其保存到组件的 ref 属性中:
```
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
componentDidMount() {
console.log(this.myRef.current);
}
render() {
return <div ref={this.myRef}>Hello, world!</div>;
}
}
```
在 componentDidMount 方法中,可以使用 this.myRef.current 获取到这个 div 元素。
2. 使用回调函数
可以将一个回调函数传递给组件,当组件挂载时,这个函数会被调用并传递组件的 DOM 节点作为参数:
```
class MyComponent extends React.Component {
componentDidMount() {
console.log(this.myNode);
}
render() {
return <div ref={node => this.myNode = node}>Hello, world!</div>;
}
}
```
在 componentDidMount 方法中,可以使用 this.myNode 获取到这个 div 元素。
需要注意的是,使用回调函数的方法在 React 16.3 版本之前是唯一的可靠方法,但是在 React 16.3 版本之后,ref 属性提供了更好的方式来获取 DOM 节点。
react获取dom的宽高
你可以使用React的`useRef`钩子来获取DOM元素的引用,然后使用`getBoundingClientRect()`方法来获取元素的宽高。
下面是一个示例代码:
```jsx
import React, { useRef } from 'react';
function MyComponent() {
const myRef = useRef(null);
function handleClick() {
const rect = myRef.current.getBoundingClientRect();
console.log(rect.width, rect.height);
}
return (
<div ref={myRef} onClick={handleClick}>
My Component
</div>
);
}
```
在上面的代码中,我们创建了一个`myRef`引用并将其分配给`<div>`元素的`ref`属性。然后,我们在点击事件处理程序中获取该元素的`getBoundingClientRect()`,并打印出其宽高。
相关推荐
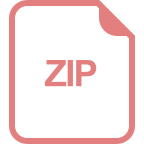
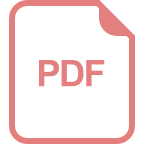
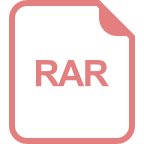
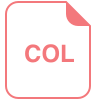
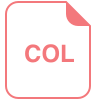
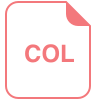
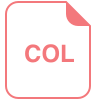
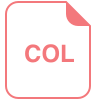







