C++ 中0X01怎么用format格式化成字符串
时间: 2024-09-19 19:02:27 浏览: 36
在C++中,如果你想将数值0X01转换成字符串并使用`std::format`格式化,你可以这样做:
```cpp
#include <iostream>
#include <format>
int main() {
int value = 0x01;
std::string str_value;
// 使用format函数将十六进制转换为字符串
std::cout << std::format("Value in decimal is {}", static_cast<int>(value)); // 输出十进制表示
std::cout << "\nValue as hexadecimal string is \"0x" + std::to_string(value) + "\""; // 转换为十六进制字符串
return 0;
}
```
在这个例子中,我们首先将整数`value`转换为整型,然后用`std::to_string`将其转换为字符串形式,以便插入到`std::format`的格式字符串中。
请注意,`std::format`在C++20及更高版本中可用,如果你的项目支持这个特性,上述代码应该可以正常工作。
相关问题
C++怎么实现整数类型转换成字符串
在C语言中,可以使用sprintf()函数将整数类型转换成字符串。sprintf()函数的原型如下:
int sprintf(char *str, const char *format, ...);
其中,str是用来存放转换后的字符串的缓冲区,format是格式化字符串,...表示可变参数列表。
例如,将整数类型变量num转换成字符串存放在buf中,可以使用以下代码:
```
int num = 123;
char buf[20];
sprintf(buf, "%d", num);
```
在上面的代码中,"%d"表示将整数类型转换成十进制整数的字符串。如果要将整数类型转换成其他进制的字符串,可以使用以下格式:
- 十六进制:"%x"或"%X"
- 八进制:"o"
- 二进制:"b"
例如,将十六进制整数类型变量num转换成字符串存放在buf中,可以使用以下代码:
```
int num = 0x123;
char buf[20];
sprintf(buf, "%X", num);
```
c++ format 例子
以下是一些C语言格式化输出的例子:
1. 输出整数:
```c
int num = 10;
printf("My favorite number is %d", num);
```
输出结果:My favorite number is 10
2. 输出浮点数:
```c
float pi = 3.1415926;
printf("The value of pi is %.2f", pi);
```
输出结果:The value of pi is 3.14
3. 输出字符:
```c
char letter = 'A';
printf("The first letter of the alphabet is %c", letter);
```
输出结果:The first letter of the alphabet is A
4. 输出字符串:
```c
char name[20] = "John";
printf("My name is %s", name);
```
输出结果:My name is John
5. 输出十六进制数:
```c
int hex = 0x2B;
printf("The value of hex is %X", hex);
```
输出结果:The value of hex is 2B
6. 输出指针地址:
```c
int *ptr = #
printf("The address of num is %p", ptr);
```
输出结果:The address of num is 0x7ffd8a5b5c9c
7. 输出多个变量:
```c
int age = 25;
float height = 1.75;
char gender = 'M';
printf("I am %d years old, %.2f meters tall, and my gender is %c", age, height, gender);
```
输出结果:I am 25 years old, 1.75 meters tall, and my gender is M
注意:在格式化输出中,%表示占位符,后面的字母表示要输出的数据类型,如%d表示输出整数,%f表示输出浮点数,%c表示输出字符,%s表示输出字符串,%X表示输出十六进制数。.2表示保留两位小数。%p表示输出指针地址。在输出多个变量时,需要按照顺序依次写出每个变量的格式。
阅读全文
相关推荐
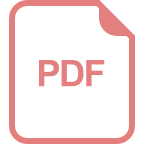
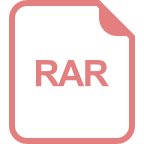
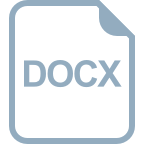
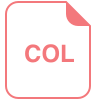
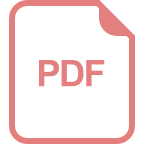
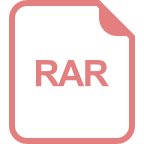
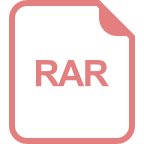
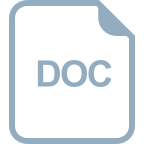
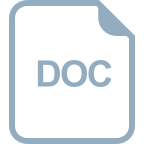
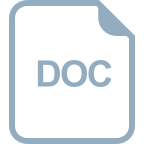
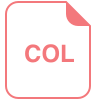
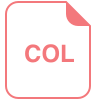
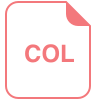
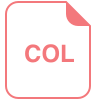
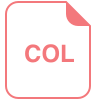
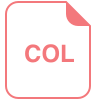
