js如何对数组进行shuffle
时间: 2024-09-20 16:13:52 浏览: 35
在JavaScript中,对数组进行随机洗牌(shuffle)通常可以使用Fisher-Yates(Knuth)算法,也称为“弹球排序”。这是一个过程,通过从数组中随机选择元素并将其位置与其后的元素交换,直到整个数组都被遍历过。以下是一个简单的实现:
```javascript
function shuffleArray(array) {
let length = array.length;
for (let i = length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1)); // 随机索引
[array[i], array[j]] = [array[j], array[i]]; // 交换元素
}
return array;
}
```
你可以像这样调用这个函数来得到一个新的随机排列的数组:
```javascript
const numbers = [1, 2, 3, 4, 5];
const shuffledNumbers = shuffleArray(numbers);
console.log(shuffledNumbers);
```
相关问题
js获取的数组随机排列
可以使用随机排序算法来实现数组的随机排列。
以下是一个简单的 JavaScript 函数,可以实现这个功能:
```js
function shuffle(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[array[i], array[j]] = [array[j], array[i]];
}
return array;
}
```
这个函数接受一个数组作为参数,然后使用 Fisher-Yates 随机排序算法对数组进行随机排序。最后返回随机排序后的数组。
使用方法如下:
```js
const myArray = [1, 2, 3, 4, 5];
const shuffledArray = shuffle(myArray);
console.log(shuffledArray); // [4, 3, 2, 1, 5](示例结果仅供参考)
```
以上代码将原始数组 `[1, 2, 3, 4, 5]` 随机排列后,结果为 `[4, 3, 2, 1, 5]`。
js打乱数组顺序的三种方法
在JavaScript中,我们可以使用几种方法来打乱数组的顺序,下面是三种常见的方法:
1. 使用Fisher-Yates算法
Fisher-Yates算法是一种常见的用于随机重新排列数组元素的算法。它逐个遍历数组的元素,并将当前元素与随机位置的元素进行交换。代码如下:
```
function shuffleArray(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[array[i], array[j]] = [array[j], array[i]];
}
return array;
}
```
2. 使用sort()方法和Math.random()
另一种方式是使用sort()方法和Math.random()生成随机数来对数组进行排序。代码如下:
```
function shuffleArray(array) {
return array.sort(() => Math.random() - 0.5);
}
```
3. 使用lodash库的shuffle()函数
如果你使用了lodash库,可以直接使用其中的shuffle()函数来对数组进行随机排序。首先,确保已经引入了lodash库,然后使用如下代码:
```
const shuffledArray = _.shuffle(array);
```
以上是三种常见的方法来打乱数组顺序,每种方法都有自己的特点和用途,请根据实际需要选择适合的方法。
阅读全文
相关推荐
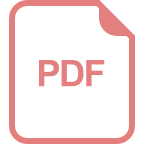
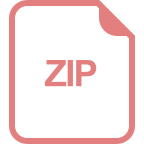
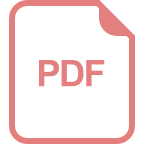
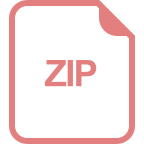
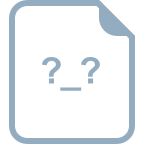
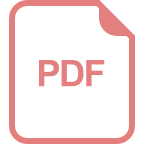
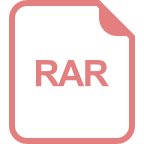
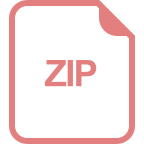
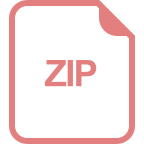
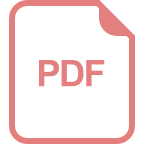
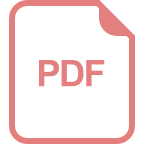
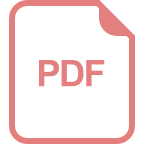
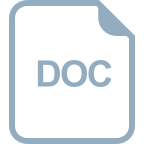
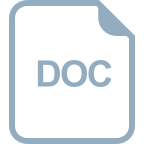
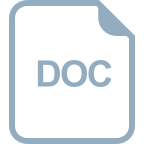
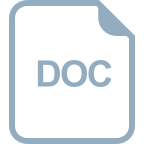
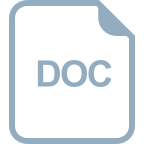