tec-xp sbb指令
时间: 2024-07-07 13:01:03 浏览: 181
Tec-Xp SBB指令是技术相关的指令集,通常用于特定的系统或编程语言中,如Teensy microcontroller platform或者一种编程环境。SBB(Subtract with Borrow)是一个二进制算术指令,它的作用是从第一个操作数中减去第二个操作数,并根据减法的结果(是否产生借位)更新低四位的状态。具体来说:
1. **含义**:SBB指令执行两个操作数的减法,并考虑借位(即如果第一个操作数小于第二个操作数,则从高位借位)。
2. **操作**:SBB指令通常涉及两个操作数,第一个作为源(source),第二个作为减数(borrow),结果存储在目标寄存器或内存位置。
3. **应用**:在编程中,SBB可用于调整数值、实现位操作,或者在计算过程中处理正负数。
相关问题
vhdl实现16位alu,可以实现加、减、与、或、非、逻辑左移、逻辑右移、算数左移、算数右移、循环左移、循环右移、和ADC/SBB指令
下面是一个简单的 VHDL 代码实现 16 位 ALU,可以支持加、减、与、或、非、逻辑左移、逻辑右移、算数左移、算数右移、循环左移、循环右移、和 ADC/SBB 指令。
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity alu_16bit is
Port ( a : in STD_LOGIC_VECTOR (15 downto 0);
b : in STD_LOGIC_VECTOR (15 downto 0);
op : in STD_LOGIC_VECTOR (3 downto 0);
carry_in : in STD_LOGIC;
result : out STD_LOGIC_VECTOR (15 downto 0);
carry_out : out STD_LOGIC;
zero : out STD_LOGIC);
end alu_16bit;
architecture Behavioral of alu_16bit is
signal temp : unsigned(16 downto 0);
signal carry : std_logic;
begin
process(a, b, op, carry_in)
begin
case op is
when "0000" => -- ADD
temp <= unsigned(a) + unsigned(b) + unsigned(carry_in);
carry <= temp(16);
result <= std_logic_vector(temp(15 downto 0));
when "0001" => -- SUB
temp <= unsigned(a) - unsigned(b) - unsigned(not carry_in);
carry <= not temp(16);
result <= std_logic_vector(temp(15 downto 0));
when "0010" => -- AND
result <= a and b;
when "0011" => -- OR
result <= a or b;
when "0100" => -- NOT
result <= not a;
when "0101" => -- LSL
result <= std_logic_vector(shift_left(unsigned(a), to_integer(unsigned(b))));
when "0110" => -- LSR
result <= std_logic_vector(shift_right(unsigned(a), to_integer(unsigned(b))));
when "0111" => -- ASL
temp <= unsigned(a) * power(2, to_integer(unsigned(b)));
carry <= temp(16);
result <= std_logic_vector(temp(15 downto 0));
when "1000" => -- ASR
temp <= shift_right(unsigned(a), to_integer(unsigned(b)));
carry <= temp(0);
result <= std_logic_vector(temp(15 downto 0));
when "1001" => -- ROL
temp <= shift_left(unsigned(a), to_integer(unsigned(b))) or shift_right(unsigned(a), 16 - to_integer(unsigned(b)));
carry <= temp(16);
result <= std_logic_vector(temp(15 downto 0));
when "1010" => -- ROR
temp <= shift_right(unsigned(a), to_integer(unsigned(b))) or shift_left(unsigned(a), 16 - to_integer(unsigned(b)));
carry <= temp(0);
result <= std_logic_vector(temp(15 downto 0));
when "1011" => -- ADC
temp <= unsigned(a) + unsigned(b) + unsigned(carry_in);
carry <= temp(16);
result <= std_logic_vector(temp(15 downto 0));
when "1100" => -- SBB
temp <= unsigned(a) - unsigned(b) - unsigned(not carry_in);
carry <= not temp(16);
result <= std_logic_vector(temp(15 downto 0));
when others => -- not a valid operation
result <= (others => 'X');
end case;
if result = (others => '0') then
zero <= '1';
else
zero <= '0';
end if;
carry_out <= carry;
end process;
end Behavioral;
```
需要注意的是,这只是一个简单的实现,可能在一些情况下需要进行修改或优化。
阅读全文
相关推荐
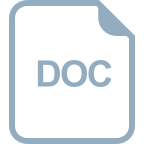
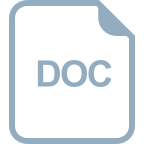
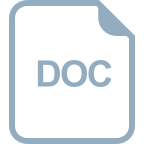
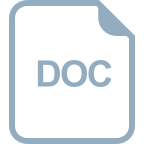
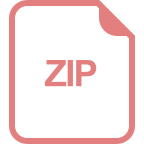
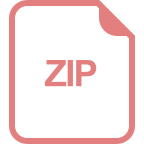
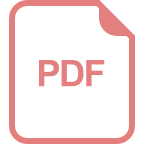
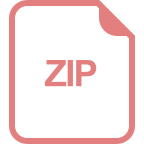
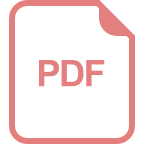
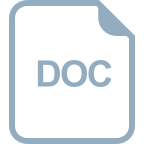
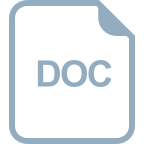
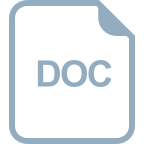
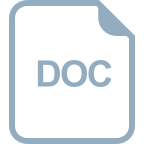
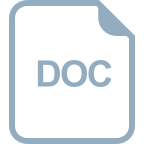
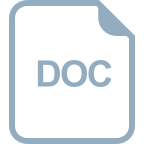