进程线程函数库C语言
时间: 2023-10-09 17:09:24 浏览: 73
在C语言中,创建线程需要使用线程函数库(pthread)。通过调用pthread_create函数来创建一个子线程,需要指定一个处理函数,该函数会在子线程中执行。创建线程的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <pthread.h>
// 子线程的处理函数
void* working(void* arg) {
printf("我是子线程,线程ID:%ld\n", pthread_self());
for(int i=0; i<9; i++) {
printf("child==i:=%d\n", i);
}
return NULL;
}
int main() {
// 创建一个子线程
pthread_t tid;
pthread_create(&tid, NULL, working, NULL);
printf("子线程创建成功,线程ID:%ld\n", tid);
// 子线程不会执行下边的代码,主线程执行
printf("我是主线程,线程ID:%ld\n", pthread_self());
for(int i=0; i<3; i++) {
printf("i=%d\n", i);
}
return 0;
}
```
在上述代码中,我们通过调用`pthread_create`函数来创建了一个子线程,并在子线程中执行了`working`函数。主线程和子线程是同时执行的,它们的执行顺序可能是不确定的。需要注意的是,主线程会在子线程之前执行完毕。
除了创建线程,线程函数库还提供了其他一些常用的函数,比如线程分离和线程取消等功能。如果需要将子线程和主线程分离,可以使用`pthread_detach`函数。示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <pthread.h>
// 子线程的处理函数
void* working(void* arg) {
printf("我是子线程,线程ID:%ld\n", pthread_self());
for(int i=0; i<9; i++) {
printf("child==i:=%d\n", i);
}
return NULL;
}
int main() {
// 创建一个子线程
pthread_t tid;
pthread_create(&tid, NULL, working, NULL);
printf("子线程创建成功,线程ID:%ld\n", tid);
// 主线程执行
printf("我是主线程,线程ID:%ld\n", pthread_self());
for(int i=0; i<3; i++) {
printf("i=%d\n", i);
}
// 设置子线程和主线程分离
pthread_detach(tid);
// 让主线程自己退出即可
pthread_exit(NULL);
return 0;
}
```
在上述代码中,我们通过调用`pthread_detach`函数将子线程和主线程分离。这样主线程执行完毕后会自动退出,不会等待子线程的结束。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C语言线程库的使用,这篇值得收藏!](https://blog.csdn.net/LxXlc468hW35lZn5/article/details/125650245)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
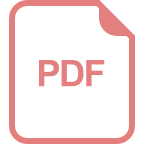
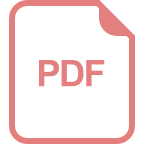
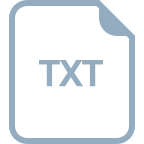














