matlab中出现m must be a vector of strictly increasing integers.怎么改正
时间: 2024-10-07 20:02:37 浏览: 17
在MATLAB中,这个错误通常表示你在尝试对向量进行操作时,遇到了不满足条件的情况。"m must be a vector of strictly increasing integers" 的意思是变量m应该是一个严格递增整数向量,即相邻元素应按顺序增加。
如果你遇到这样的错误,你可以按照以下几个步骤检查并修正:
1. **检查数据类型**:确认`m`是否真的是一维整数向量,而不是其他类型的数据,如字符串、复数等。
2. **检查数值顺序**:确保所有元素是连续的,并且没有重复的值。如果有非递增的元素,你需要调整它们。
3. **检查长度**:如果`m`不是严格的递增序列,你可能需要删除多余的元素或填充正确的值。
4. **代码审查**:查看代码中涉及`m`的部分,看是否有哪里误用了它,例如可能是期望它是排序后的数组但实际传入了未排序的。
5. **示例错误修复**:
```matlab
% 假设原来的m有问题
m = [1 3 2]; % 这不是一个递增的向量,因为3不应该在1之前
% 修正
m = sort(m); % 对m进行升序排序
```
相关问题
ValueError: Each lower bound must be strictly less than each upper bound.
这个错误通常是因为你在使用某些函数或方法时,传递了一个范围(上下界),但是上下界的值不符合要求。一般来说,下界的值应该小于上界的值。
以下是一个例子:
```
import numpy as np
# 创建一个范围(上下界)数组
bounds = np.array([[-1.0, 1.0], [-2.0, 2.0], [0.0, 5.0]])
# 尝试创建一个随机样本
sample = np.random.uniform(bounds[:, 0], bounds[:, 1])
# 如果上下界的值不符合要求,将会抛出 ValueError 错误
# 例如,如果我们将下界设为大于上界,就会出现这个错误
bounds = np.array([[1.0, -1.0], [-2.0, 2.0], [0.0, 5.0]])
sample = np.random.uniform(bounds[:, 0], bounds[:, 1])
```
在这个例子中,我们试图创建一个在给定范围内的随机样本。首先,我们创建一个范围数组 `bounds`,然后使用 `np.random.uniform()` 函数生成一个随机样本。如果 `bounds` 数组中的上下界符合要求,那么这个函数就能够正常工作。但是,如果我们将下界设为大于上界,就会出现 `ValueError: Each lower bound must be strictly less than each upper bound` 错误。因此,我们需要确保 `bounds` 数组中的上下界符合要求。
mysql创建分区表时报这个错什么意思 VALUES LESS THAN value must be strictly increasing for each partition
这个错误的意思是在创建MySQL分区表时,你所定义的分区值(VALUES LESS THAN value)必须是严格递增的。也就是说每个分区的值都必须大于上一个分区的值,这是MySQL分区表的规则之一。
相关推荐
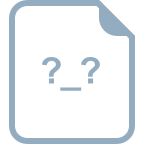
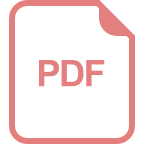













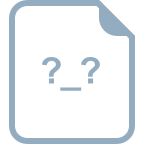
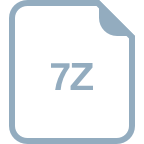