在spring MVC中使用webclient的最佳实践
时间: 2024-09-14 20:12:25 浏览: 54
在Spring MVC中,WebClient是一个强大的工具,用于RESTful API的客户端操作。以下是使用WebClient的一些最佳实践:
1. **依赖注入**:通常会在Spring配置文件中通过`@Autowired`将WebClient注入到需要它的组件(如Service或Repository),这样可以更好地管理生命周期。
```java
@Autowired
private WebClient webClient;
```
2. **工厂模式**:创建WebClient实例时,可以使用工厂模式封装配置细节,如超时、重试策略等,提高代码复用性和可维护性。
```java
public class WebClientFactory {
public WebClient createWebClient() {
return WebClient.builder().baseUrl("http://api.example.com").build();
}
}
```
3. **使用断言处理**:发送请求后,可以使用`ExchangeStrategies`和`ResponseSpec`来验证响应,如检查HTTP状态码、内容类型等。
```java
Mono<ServerResponse> response = webClient.get()
.uri("/users/{id}", id)
.headers(h -> h.accept(MediaType.APPLICATION_JSON))
.exchange(ClientResponse.BUILDER);
response
.expectStatus(isOk())
.expectBody(String.class).isEqualTo("expected result");
```
4. **错误处理和异常处理**:使用try-catch块处理可能出现的网络异常和API返回错误。
```java
try {
ServerResponse userResponse = webClient.get().uri("/{username}")
.retrieve().bodyToMono(User.class);
} catch (HttpClientErrorException e) {
// Handle error code and message
}
```
5. **批量请求和并发控制**:可以利用WebClient的流式API进行并行请求,使用`parallelStream()`或`collectList()`方法。
6. **线程安全**:如果在多线程环境中使用,确保WebClient实例是线程安全的,可以通过单例模式或使用WebClient.Builder的withSession方法。
阅读全文
相关推荐
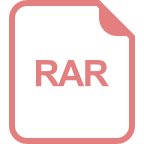
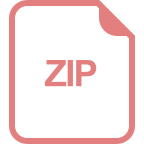

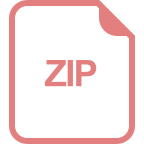
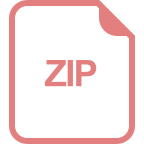
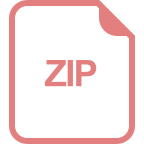
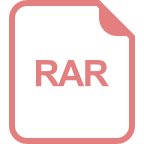
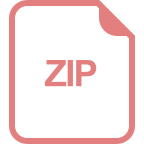
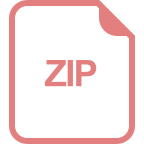
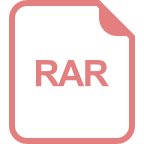
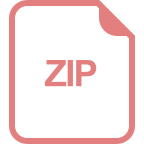
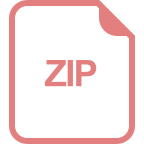
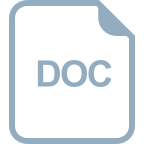
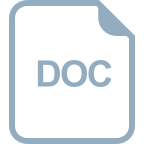
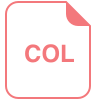
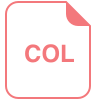



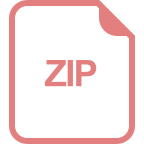