java编程多态练习停车场收费系统
时间: 2023-12-11 19:03:43 浏览: 31
以下是Java编程实现停车场收费系统的多态练习题解答:
```java
// 定义车辆类
class Vehicle {
protected String type; // 车型
protected int hours; // 停车时间
public Vehicle(String type, int hours) {
this.type = type;
this.hours = hours;
}
// 计算停车费用
public double calculateFee() {
return 0;
}
}
// 定义客车类
class Bus extends Vehicle {
public Bus(int hours) {
super("客车", hours);
}
// 重写计算停车费用方法
public double calculateFee() {
return hours * 15;
}
}
// 定义货车类
class Truck extends Vehicle {
public Truck(int hours) {
super("货车", hours);
}
// 重写计算停车费用方法
public double calculateFee() {
return hours * 12;
}
}
// 定义轿车类
class Car extends Vehicle {
public Car(int hours) {
super("轿车", hours);
}
// 重写计算停车费用方法
public double calculateFee() {
return hours * 8;
}
}
// 定义停车场类
class ParkingLot {
private Vehicle[] vehicles; // 车辆数组
private int count; // 车辆数量
public ParkingLot() {
vehicles = new Vehicle[100];
count = 0;
}
// 添加车辆
public void addVehicle(Vehicle vehicle) {
vehicles[count++] = vehicle;
}
// 计算总停车费用
public double calculateTotalFee() {
double totalFee = 0;
for (int i = 0; i < count; i++) {
totalFee += vehicles[i].calculateFee();
}
return totalFee;
}
}
// 测试类
public class Test {
public static void main(String[] args) {
ParkingLot parkingLot = new ParkingLot();
parkingLot.addVehicle(new Bus(2));
parkingLot.addVehicle(new Truck(3));
parkingLot.addVehicle(new Car(4));
System.out.println("总停车费用为:" + parkingLot.calculateTotalFee()); // 输出:总停车费用为:119.0
}
}
```
相关推荐
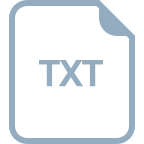
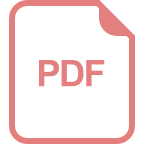
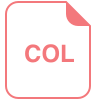
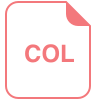
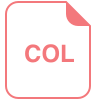
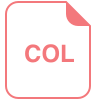
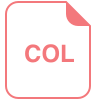









