计算属性computed购物车
时间: 2023-11-15 13:03:24 浏览: 105
计算属性computed在购物车中可以用来计算商品的总价或者总数量。它是Vue.js提供的一种便捷的属性计算方式,可以根据已有的数据计算出新的属性值,而且计算结果会被缓存起来,只有在依赖的数据发生变化时才会重新计算。在购物车中,我们可以使用computed来计算选中商品的总价或者总数量,从而方便地展示给用户。
举个例子,假设我们有一个购物车列表,每个商品都有数量和单价两个属性,我们可以使用computed来计算选中商品的总价:
```
computed: {
totalPrice() {
let total = 0;
this.cartList.forEach(item => {
if (item.checked) {
total += item.quantity * item.price;
}
});
return total;
}
}
```
在上面的代码中,我们定义了一个名为totalPrice的计算属性,它会遍历购物车列表中的每个商品,如果该商品被选中了(即checked属性为true),就将该商品的数量乘以单价累加到总价中。最后返回总价即可。
相关问题
使用计算属性完成购物车功能
当使用Vue.js开发购物车功能时,可以使用计算属性来实现购物车的逻辑。计算属性是在Vue实例中定义的一个属性,其值根据其他数据的变化而变化,类似于响应式的计算结果。
下面是一个简单的购物车示例,展示如何使用计算属性完成购物车功能:
```html
<!DOCTYPE html>
<html>
<head>
<title>购物车</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h2>购物车</h2>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }} - ¥{{ item.price }}
<button @click="addToCart(item)">加入购物车</button>
</li>
</ul>
<h3>购物车内容:</h3>
<ul>
<li v-for="cartItem in cartItems" :key="cartItem.id">
{{ cartItem.name }} - ¥{{ cartItem.price }}
</li>
</ul>
<p>总价:¥{{ total }}</p>
</div>
<script>
new Vue({
el: "#app",
data: {
items: [
{ id: 1, name: "商品1", price: 100 },
{ id: 2, name: "商品2", price: 200 },
{ id: 3, name: "商品3", price: 300 }
],
cart: []
},
computed: {
cartItems() {
// 根据购物车中的商品id,从商品列表中获取对应的商品信息
return this.items.filter(item => this.cart.includes(item.id));
},
total() {
// 计算购物车中商品的总价
return this.cartItems.reduce((total, item) => total + item.price, 0);
}
},
methods: {
addToCart(item) {
// 将商品id添加到购物车数组中
this.cart.push(item.id);
}
}
});
</script>
</body>
</html>
```
在这个示例中,我们使用Vue.js构建了一个简单的购物车功能。商品列表中包含了三个商品,每个商品都可以点击"加入购物车"按钮将其添加到购物车中。计算属性`cartItems`根据购物车数组中的商品id,在商品列表中过滤出对应的商品信息。计算属性`total`根据购物车中的商品信息计算出总价。
请注意,这只是一个简单的示例,实际的购物车功能可能涉及到更复杂的逻辑,例如数量的增减、删除商品等。这个示例可以作为一个起点来为你的购物车功能添加更多的功能和业务逻辑。
利用vue搭建商品列表并应用计算属性统计购物车中的商品总价程序
好的,下面是一个简单的利用Vue搭建商品列表并应用计算属性统计购物车中的商品总价的程序:
HTML代码:
```
<div id="app">
<h2>商品列表</h2>
<ul>
<li v-for="item in products" :key="item.id">
{{ item.name }} - {{ item.price }}元
<button @click="addToCart(item)">加入购物车</button>
</li>
</ul>
<h2>购物车</h2>
<ul>
<li v-for="item in cartItems" :key="item.id">
{{ item.name }} - {{ item.price }}元 x {{ item.quantity }}
<button @click="removeFromCart(item)">移出购物车</button>
</li>
</ul>
<p>总价:{{ totalPrice }}元</p>
</div>
```
JavaScript代码:
```
var app = new Vue({
el: '#app',
data: {
products: [
{ id: 1, name: '商品1', price: 10 },
{ id: 2, name: '商品2', price: 20 },
{ id: 3, name: '商品3', price: 30 }
],
cartItems: []
},
methods: {
addToCart: function(item) {
var cartItem = this.cartItems.find(function(cartItem) {
return cartItem.id === item.id;
});
if (cartItem) {
cartItem.quantity++;
} else {
this.cartItems.push({
id: item.id,
name: item.name,
price: item.price,
quantity: 1
});
}
},
removeFromCart: function(item) {
var index = this.cartItems.indexOf(item);
this.cartItems.splice(index, 1);
}
},
computed: {
totalPrice: function() {
var total = 0;
this.cartItems.forEach(function(item) {
total += item.price * item.quantity;
});
return total;
}
}
});
```
解释:
1. 首先定义了一个Vue实例,将其挂载到id为`app`的DOM元素上。
2. 在Vue实例的data属性中定义了两个数组变量:`products`表示商品列表,`cartItems`表示购物车中的商品。
3. 在Vue实例的methods属性中定义了两个方法:`addToCart`用于将商品加入购物车中,`removeFromCart`用于将商品从购物车中移除。
4. 在Vue实例的computed属性中定义了一个计算属性`totalPrice`,用于计算购物车中的商品总价。
5. 在HTML中,使用Vue的指令`v-for`展示商品列表和购物车中的商品,使用Vue的指令`v-on`绑定点击事件。
6. 在HTML中,使用Vue的插值语法`{{}}`展示商品名称、商品价格、商品数量和商品总价。
这个程序可以实现一个简单的购物车功能,你可以根据自己的需求进行改进。
阅读全文
相关推荐


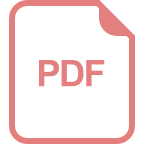











