用C语言写出递归方法实现对一个链表的值进行正逆序输出ABCCBA
时间: 2024-10-07 15:05:43 浏览: 31
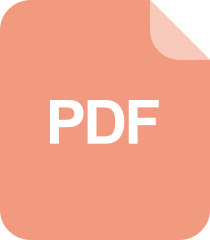
C语言实现单链表逆序与逆序输出实例
在C语言中,我们可以使用递归函数来实现链表值的正序和逆序输出。首先,我们需要定义链表结构体和一些辅助函数,比如打印节点值、获取下一个节点等。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 打印节点数据
void printNode(Node* node) {
printf("%d ", node->data);
}
// 递归遍历并打印链表
void printListRecursively(Node* head, char order) {
if (head == NULL) {
return; // 如果链表为空,返回
}
if (order == 'N') { // 逆序输出
printNode(head);
printListRecursively(head->next, order); // 递归处理下一个节点
} else { // 正序输出
printListRecursively(head->next, order); // 先处理下一个节点
printNode(head); // 最后打印当前节点
}
}
int main() {
// 创建链表示例: A -> B -> C -> C -> B -> A
Node* list = createNode('A');
list->next = createNode('B');
list->next->next = createNode('C');
list->next->next->next = createNode('C');
list->next->next->next->next = createNode('B');
list->next->next->next->next->next = createNode('A');
// 输出正序和逆序
printf("正序输出: ");
printListRecursively(list, 'F'); // F表示正序(Forward)
printf("\n逆序输出: ");
printListRecursively(list, 'N'); // N表示逆序(Reverse)
return 0;
}
```
在这个示例中,`printListRecursively` 函数会根据传入的 `order` 参数决定是按正序还是逆序输出。
阅读全文
相关推荐
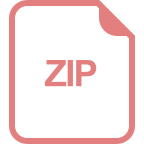
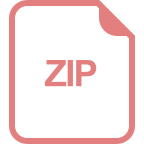



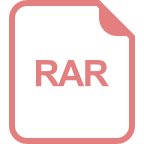
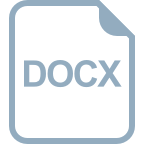
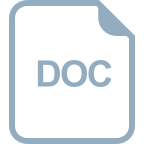
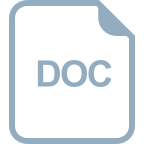







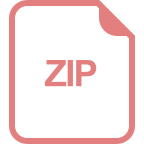