设计一个程序,输入按降序排序的5个学生的考试成绩,保存在数组中,输入一个成绩,对数组中的成绩进行二分查找,如查找到,输出查询的分数及名次,如果没有查找到,只输出-1。c语言
时间: 2024-12-25 10:26:20 浏览: 3
设计一个C语言程序,首先我们需要定义一个结构体来存储学生信息,包括姓名和成绩。然后创建一个包含这五个学生成绩的动态数组,按照降序排列。接下来编写一个函数来进行二分查找。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct {
char name[20];
int score;
} Student;
// 按照成绩降序排序
int compare(const void* a, const void* b) {
return ((const Student*)b)->score - ((const Student*)a)->score;
}
// 动态分配并初始化学生数组
void init_students(Student** arr, int size, int scores[]) {
for (int i = 0; i < size; i++) {
arr[i] = (Student*)malloc(sizeof(Student));
scanf("%s %d", arr[i]->name, &arr[i]->score);
// 先将所有元素插入,后续再进行降序排序
*(scores + i) = arr[i]->score;
}
qsort(arr, size, sizeof(Student), compare);
}
// 二分查找函数
int binary_search(Student* arr, int n, int target_score) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid].score == target_score)
return mid + 1; // 返回分数和名次(从1开始计数)
else if (arr[mid].score > target_score)
right = mid - 1;
else
left = mid + 1;
}
return -1; // 如果没找到,则返回-1
}
int main() {
int scores[5];
Student* students;
int size = 5;
init_students(&students, size, scores);
int search_score;
printf("请输入你要查找的成绩:");
scanf("%d", &search_score);
int result = binary_search(students, size, search_score);
if (result != -1) {
printf("找到了,分数:%d 名次:%d\n", search_score, result);
} else {
printf("未找到该成绩,结果:-1\n");
}
// 清理内存
for (int i = 0; i < size; i++)
free(students[i]);
free(students);
return 0;
}
```
阅读全文
相关推荐
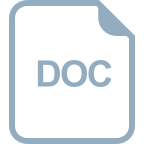
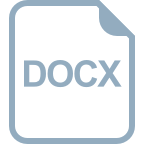
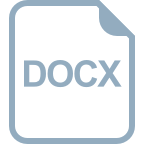
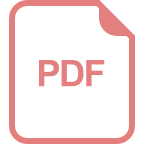
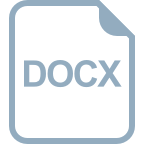
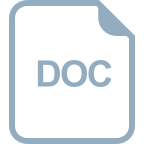
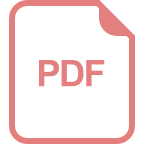
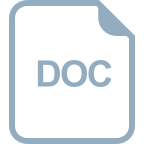
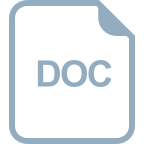
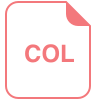
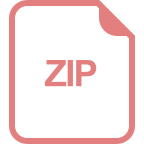
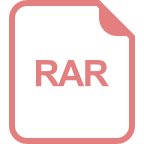
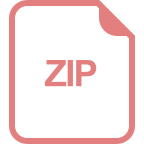
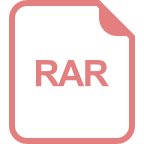
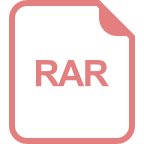