int CR_RegisterEventCallback(int nDetrIdx, ICallback∗ pCallback ) ; class ICallback { public: ICallback () {}; virtual ˜ ICallback () {}; void virtual Process (int nEventID, CR Event∗ pEvent) {}; }; enum CR EventID { CR EVT SERVER DISCONNECTED, // dropped connection with server CR EVT DETR DISCONNECTED, // dropped connection with detector CR EVT TEMPERATURE INFO, // temperature of the detector CR EVT NEW FRAME, // Arrival of a new frame CR EVT CALIBRATION IN PROGRESS, // Calibration in progress CR EVT CALIBRATION FINISHED, // Completion of calibration CR EVT ACQ STAT INFO // Acquisition of statistical summary }; struct CR Event { int nDetrIdx; int nWidth; // Same as CR ModeInfo.nImageWidth, see A.4 int nHeight; int nPixelDepth; void∗ pData; }; struct CR AcquisitionStatInfo { int nTotalFrameNum; // Total number of frames acquired int nLostFrameNum; // Number of lost frames flfloat fStatFrameRate; flfloat fTransmissionSpeed; long long nAcqDuration; // Duration of image acquisition };python调用
时间: 2024-04-14 16:25:39 浏览: 82
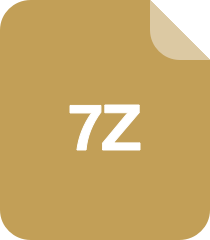
aidltest.7z adilClient adilServer
要在Python中调用这段C++代码,你需要使用ctypes库来实现。
首先,我们需要将C++中的枚举类型和结构体在Python中进行定义。以下是一个示例代码:
```python
import ctypes
# 定义枚举类型
class CREventID(ctypes.c_int):
CR_EVT_SERVER_DISCONNECTED = 0
CR_EVT_DETR_DISCONNECTED = 1
CR_EVT_TEMPERATURE_INFO = 2
CR_EVT_NEW_FRAME = 3
CR_EVT_CALIBRATION_IN_PROGRESS = 4
CR_EVT_CALIBRATION_FINISHED = 5
CR_EVT_ACQ_STAT_INFO = 6
# 定义结构体
class CREvent(ctypes.Structure):
_fields_ = [
("nDetrIdx", ctypes.c_int),
("nWidth", ctypes.c_int),
("nHeight", ctypes.c_int),
("nPixelDepth", ctypes.c_int),
("pData", ctypes.c_void_p),
]
class CRAcquisitionStatInfo(ctypes.Structure):
_fields_ = [
("nTotalFrameNum", ctypes.c_int),
("nLostFrameNum", ctypes.c_int),
("fStatFrameRate", ctypes.c_float),
("fTransmissionSpeed", ctypes.c_float),
("nAcqDuration", ctypes.c_longlong),
]
```
然后,我们可以加载C++动态链接库,并设置函数的参数类型和返回类型。以下是一个示例代码:
```python
# 加载C++动态链接库
mylib = ctypes.cdll.LoadLibrary("your_cpp_library.so")
# 定义函数参数类型和返回类型
mylib.CR_RegisterEventCallback.argtypes = [ctypes.c_int, ctypes.POINTER(ICallback)]
mylib.CR_RegisterEventCallback.restype = ctypes.c_int
```
接下来,我们可以创建回调函数的实例,并将其传递给`CR_RegisterEventCallback`函数进行注册。
最后,我们可以调用C++函数并传递相应的参数。
请确保在Python代码中正确加载和调用C++动态链接库,并根据实际情况设置函数参数类型和返回类型。根据你的实际需求,可能需要调整代码以适应你的情况。
阅读全文
相关推荐
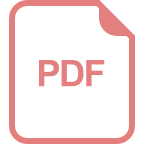
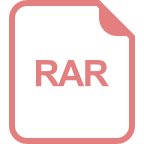



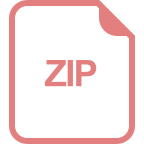