phyton圣诞树粒子代码
时间: 2024-12-06 21:16:00 浏览: 27
Python 中创建圣诞树形状的粒子效果通常涉及到基本的图形操作和循环结构。这里简单提供一个使用 ASCII 码构建一个简单的动态圣诞树的例子,你可以通过控制循环次数、字符等变量来调整效果。
```python
def print_christmas_tree(n):
for i in range(n):
# 基本的树干部分
if i == 0 or i == n - 1:
print('#' * (i + 1))
else:
# 树枝部分
print(' ' * (n - i - 1) + '#' + ' ' * (2 * i - 1) + '#')
# 粒子装饰
if i % 2 == 0: # 如果是偶数行,添加一些星号作为装饰
print('*' * (2 * i))
# 打印圣诞树
print_christmas_tree(5)
```
在这个代码里,`print_christmas_tree()` 函数接受一个整数 `n`,表示树的高度。通过循环和条件判断,生成了类似于下面这样的圣诞树:
```
####
***
*****
*******
* *
```
如果你想让这看起来更像“粒子”,可以尝试加入更多的动态元素,如随机移动或颜色变化,但这已经超出了基础ASCII艺术的范围,需要引入更复杂的库或者游戏引擎。
相关问题
python圣诞树粒子代码
以下是一个使用Python的turtle模块绘制圣诞树粒子的例子:
```python
import turtle
def draw_tree():
turtle.speed(10)
turtle.penup()
turtle.goto(0, -200)
turtle.pendown()
turtle.color("green")
turtle.begin_fill()
turtle.left(90)
turtle.forward(200)
turtle.right(135)
turtle.forward(280)
turtle.left(135)
turtle.forward(280)
turtle.right(135)
turtle.forward(200)
turtle.end_fill()
def draw_particle(x, y):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.color("red")
turtle.begin_fill()
turtle.circle(5)
turtle.end_fill()
def main():
turtle.setup(800, 600)
turtle.bgcolor("black")
turtle.title("Christmas Tree Particle")
turtle.hideturtle()
turtle.speed(0)
draw_tree()
for _ in range(100):
x = random.randint(-400, 400)
y = random.randint(-200, 200)
draw_particle(x, y)
turtle.done()
if __name__ == "__main__":
main()
```
Python圣诞树粒子代码
Python圣诞树粒子代码通常用于创建交互式的圣诞树效果,通过控制小点(也称为“粒子”)的移动、颜色变化等动画行为,让屏幕上的圣诞树看起来更生动有趣。下面是一个简单的例子,使用PIL库(Python Imaging Library)来绘制:
```python
import random
from PIL import Image, ImageDraw
# 定义圣诞树形状
def draw_tree(draw, x, y, size=50):
for angle in range(0, 360, 30):
dx = int(size * (2 + cos(angle))) * cos(angle)
dy = int(size * (2 + cos(angle))) * sin(angle)
draw.line([(x+dx, y+dy), (x, y)], fill='green', width=size//4)
# 创建画布和画笔
image_size = (500, 500)
img = Image.new('RGB', image_size, color='white')
draw = ImageDraw.Draw(img)
# 主体部分:圣诞树
x, y = image_size[0] // 2, image_size[1] // 3
draw_tree(draw, x, y)
# 颗粒(粒子)
particle_count = 100
particles = [(random.randint(x//2, image_size[0]-x//2), random.randint(y//2, y*3)) for _ in range(particle_count)]
for particle in particles:
# 绘制粒子
draw.ellipse([particle[0] - 3, particle[1] - 3, particle[0] + 3, particle[1] + 3], fill='red')
# 显示和保存结果
img.show()
img.save('christmas_tree_particles.png')
阅读全文
相关推荐
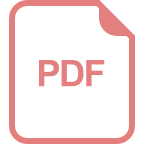













