package main import ( "bytes" "encoding/json" "fmt" "net/http" "github.com/gin-gonic/gin" ) type AlertData struct { Receiver string `json:"receiver"` Status string `json:"status"` Alerts []Alert `json:"alerts"` GroupLabels map[string]string `json:"groupLabels"` CommonLabels map[string]string `json:"commonLabels"` CommonAnnotations map[string]string `json:"commonAnnotations"` ExternalURL string `json:"externalURL"` } type Alert struct { Status string `json:"status"` Labels map[string]string `json:"labels"` Annotations map[string]string `json:"annotations"` } func main() { router := gin.Default() router.POST("/webhook", handleWebhook) router.Run(":8080") } func handleWebhook(c *gin.Context) { var alertData AlertData err := c.BindJSON(&alertData) if err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": "Error decoding JSON"}) return } // Process the received alert data for _, alert := range alertData.Alerts { // Extract information from alert alertName := alert.Labels["alertname"] instance := alert.Labels["instance"] severity := alert.CommonLabels["severity"] description := alert.Annotations["description"] summary := alert.Annotations["summary"] // Compose the message to be sent to Enterprise WeChat group using Markdown format message := fmt.Sprintf(`**Alert Name:** %s **Instance:** %s **Severity:** %s **Description:** %s **Summary:** %s`, alertName, instance, severity, description, summary) // Send the message to Enterprise WeChat group using the WeChat bot API sendToEnterpriseWeChatGroup(message) } c.JSON(http.StatusOK, gin.H{"message": "Alerts processed successfully"}) } func sendToEnterpriseWeChatGroup(message string) { // Replace 'YOUR_WECHAT_BOT_URL' with the actual URL of your Enterprise WeChat bot wechatBotURL := "YOUR_WECHAT_BOT_URL" data := map[string]interface{}{ "msgtype": "markdown", "markdown": map[string]string{ "content": message, }, } jsonData, _ := json.Marshal(data) _, err := http.Post(wechatBotURL, "application/json", bytes.NewReader(jsonData)) if err != nil { fmt.Println("Error sending message to Enterprise WeChat group:", err) } } 将以上代码拆分成多个模块
时间: 2024-01-11 12:04:06 浏览: 26
将以上代码拆分为多个模块,可以按照以下方式进行组织:
**main.go:**
```go
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
router := gin.Default()
router.POST("/webhook", handleWebhook)
router.Run(":8080")
}
```
**alert.go:**
```go
package main
type AlertData struct {
Receiver string `json:"receiver"`
Status string `json:"status"`
Alerts []Alert `json:"alerts"`
GroupLabels map[string]string `json:"groupLabels"`
CommonLabels map[string]string `json:"commonLabels"`
CommonAnnotations map[string]string `json:"commonAnnotations"`
ExternalURL string `json:"externalURL"`
}
type Alert struct {
Status string `json:"status"`
Labels map[string]string `json:"labels"`
Annotations map[string]string `json:"annotations"`
}
```
**webhook.go:**
```go
package main
import (
"bytes"
"encoding/json"
"fmt"
"github.com/gin-gonic/gin"
"net/http"
)
func handleWebhook(c *gin.Context) {
var alertData AlertData
err := c.BindJSON(&alertData)
if err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": "Error decoding JSON"})
return
}
// Process the received alert data
for _, alert := range alertData.Alerts {
// Extract information from alert
alertName := alert.Labels["alertname"]
instance := alert.Labels["instance"]
severity := alert.CommonLabels["severity"]
description := alert.Annotations["description"]
summary := alert.Annotations["summary"]
// Compose the message to be sent to Enterprise WeChat group using Markdown format
message := fmt.Sprintf(`**Alert Name:** %s
**Instance:** %s
**Severity:** %s
**Description:** %s
**Summary:** %s`,
alertName, instance, severity, description, summary)
// Send the message to Enterprise WeChat group using the WeChat bot API
sendToEnterpriseWeChatGroup(message)
}
c.JSON(http.StatusOK, gin.H{"message": "Alerts processed successfully"})
}
```
**wechat.go:**
```go
package main
import (
"bytes"
"encoding/json"
"fmt"
"net/http"
)
func sendToEnterpriseWeChatGroup(message string) {
// Replace 'YOUR_WECHAT_BOT_URL' with the actual URL of your Enterprise WeChat bot
wechatBotURL := "YOUR_WECHAT_BOT_URL"
data := map[string]interface{}{
"msgtype": "markdown",
"markdown": map[string]string{
"content": message,
},
}
jsonData, _ := json.Marshal(data)
_, err := http.Post(wechatBotURL, "application/json", bytes.NewReader(jsonData))
if err != nil {
fmt.Println("Error sending message to Enterprise WeChat group:", err)
}
}
```
通过将不同功能的代码拆分到不同的模块中,可以更好地组织代码,提高代码的可读性和可维护性。在`main.go`中导入其他模块,并在适当的地方调用相应的函数即可。
相关推荐
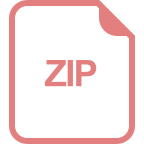
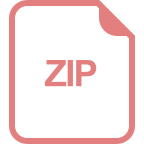
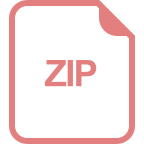















