用Python文件拷贝
时间: 2024-05-24 10:08:18 浏览: 68
在Python中,你可以使用shutil模块来拷贝文件。shutil模块提供了很多高级的文件操作功能,其中之一就是拷贝文件。你可以使用shutil.copy()函数来实现文件的拷贝,该函数的语法如下:
shutil.copy(src, dst)
其中,src是源文件的路径,dst是目标文件的路径。这个函数会将源文件拷贝到目标文件中。如果目标文件已经存在,则会被覆盖。如果目标文件的路径不存在,则会抛出异常。
例如,你可以使用以下代码将一个名为file1.txt的文件从源目录拷贝到目标目录:
```
import shutil
src = '/path/to/source/file1.txt'
dst = '/path/to/destination/file1.txt'
shutil.copy(src, dst)
```
如果你想拷贝整个目录,可以使用shutil.copytree()函数。例如,你可以使用以下代码将一个名为dir1的目录从源目录拷贝到目标目录:
```
import shutil
src = '/path/to/source/dir1'
dst = '/path/to/destination/dir1'
shutil.copytree(src, dst)
```
相关问题
python文件拷贝
要在 Python 中拷贝文件,你可以使用 `shutil` 模块的 `copy` 函数。下面是一个示例代码,演示如何拷贝一个文件:
```python
import shutil
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file.txt'
shutil.copy(source_file, destination_file)
```
请确保将 `source_file` 替换为源文件的路径,将 `destination_file` 替换为目标文件的路径。这样就可以将源文件拷贝到目标路径中了。如果目标路径中已经存在同名文件,它将会被覆盖。如果你想保留文件元数据(如访问时间、修改时间等),可以使用 `shutil.copy2` 函数。
希望这可以帮助到你!如果你还有其他问题,请随时提问。
python 文件拷贝
你可以使用 Python 的 shutil 模块来复制文件。具体的操作可以按照以下步骤进行:
```python
import shutil
# 定义源文件和目标文件的路径
source_file = 'path/to/source_file.txt'
target_file = 'path/to/target_file.txt'
# 执行文件拷贝操作
shutil.copy(source_file, target_file)
```
在上述示例中,你需要将 `path/to/source_file.txt` 替换为源文件的实际路径,将 `path/to/target_file.txt` 替换为目标文件的实际路径。这样,源文件就会被复制到目标文件所在的位置。
值得注意的是,如果目标文件已经存在,该操作会覆盖目标文件。如果你想保留原始文件的权限和其他元数据,可以使用 `shutil.copy2()` 方法代替 `shutil.copy()` 方法。
阅读全文
相关推荐
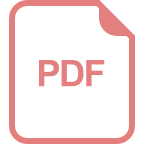
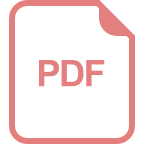
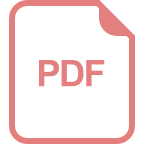
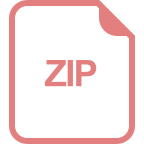












