用c语言的seqlist(序列列表)数据结构实现二进制数的加一操作?
时间: 2024-11-27 11:18:31 浏览: 9
在C语言中,我们可以使用链表(Sequence List,这里的`seqlist`通常指的是动态数组或单向链表)数据结构来表示二进制数。为了实现二进制数的加一操作,我们需要维护一个`Node`结构体,每个节点代表二进制位,并有前驱和后继指针。
首先,创建一个`Node`结构:
```c
typedef struct Node {
int value; // 二进制位值
struct Node* next; // 指向前一个位或NULL(表示最高位)
} Node;
```
然后,我们可以定义一个`BinaryList`结构,包含头结点指针:
```c
typedef struct BinaryList {
Node* head;
int length;
} BinaryList;
```
接下来,我们编写函数来添加一位到二进制数:
1. 初始化函数(如果链表为空):
```c
void add_one(BinaryList* list) {
if (list->head == NULL) {
list->head = list->tail = create_new_node(1); // 初始值为1
list->length = 1;
}
}
```
2. 添加一位并处理进位:
```c
void increment(BinaryList* list) {
Node* current = list->head;
while (current != NULL) {
if (current->value == 0) {
current->value = 1;
if (current->next == NULL) { // 如果这是最高位
// 创建新节点并将当前位设为0(相当于清零),并处理下一位的进位
Node* new_head = create_new_node(0);
new_head->next = current->next;
list->head = new_head;
current->next = NULL;
} else {
break; // 进入下一位
}
} else {
current->value++;
// 如果当前位超过1,则需要进位(即将当前位置0,下一位+1)
if (current->value > 1) {
current->value = 0;
current->next = create_new_node(1); // 新增一位
}
break;
}
current = current->next;
}
}
```
3. 辅助函数`create_new_node`用于创建新的二进制位节点:
```c
Node* create_new_node(int value) {
Node* newNode = malloc(sizeof(Node));
newNode->value = value;
newNode->next = NULL;
return newNode;
}
```
最后,记得释放不再使用的内存。这个实现假设链表中存储的是从最低有效位(LSB)开始的二进制数。
阅读全文
相关推荐




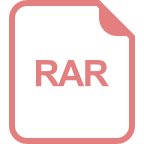
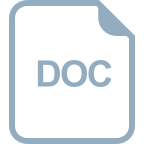












