Katalon利用java-diff-utils库,写一个Groovy脚本:自动比较两个文件夹下的大数据量的同名文件,并将比较结果以HTML表格的形式输出成html文件。 具体要求:当文件只在其中一个文件夹中存在时,将该信息输出到html文件中; 当文件在两个文件夹中都存在时,将两边文件中的差异行数及差异内容都出力到html文件中,并将有差异的字段以黄色背景色高亮显示出来,并且能识别出隔行数据。
时间: 2024-04-12 18:28:04 浏览: 15
您可以使用以下Groovy脚本来实现此功能:
```groovy
@Grab(group='com.googlecode.java-diff-utils', module='diffutils', version='1.3.0')
import difflib.DiffUtils
import difflib.Patch
import groovy.xml.MarkupBuilder
// 定义文件夹路径
def folder1Path = "/path/to/folder1"
def folder2Path = "/path/to/folder2"
// 创建HTML表格
def createHtmlTable(diffLines, writer) {
writer.html {
body {
table(border: "1") {
diffLines.each { line ->
tr {
line.each { cell ->
td {
if (cell.startsWith("+")) {
span(style: "background-color: yellow") {
text(cell.drop(1))
}
} else if (cell.startsWith("-")) {
span(style: "background-color: yellow") {
strike {
text(cell.drop(1))
}
}
} else {
text(cell)
}
}
}
}
}
}
}
}
}
// 比较两个文件的差异
def compareFiles(file1, file2, writer) {
def lines1 = file1.readLines()
def lines2 = file2.readLines()
def patch = DiffUtils.diff(lines1, lines2)
def diffLines = patch.getDeltas().collect { delta ->
delta.getOriginal().getLines() + delta.getRevised().getLines()
}
createHtmlTable(diffLines, writer)
}
// 比较文件夹中的同名文件
def compareFolders(folder1, folder2, writer) {
def files1 = folder1.listFiles()
def files2 = folder2.listFiles()
files1.each { file1 ->
def matchingFile = files2.find { file2 -> file2.name == file1.name }
if (matchingFile) {
compareFiles(file1, matchingFile, writer)
} else {
writer.p {
text("File only exists in folder 1: ${file1.name}")
}
}
}
files2.each { file2 ->
def matchingFile = files1.find { file1 -> file1.name == file2.name }
if (!matchingFile) {
writer.p {
text("File only exists in folder 2: ${file2.name}")
}
}
}
}
// 执行比较并输出为HTML文件
def outputFile = new File("/path/to/output.html")
outputFile.withWriter { writer ->
def xml = new MarkupBuilder(writer)
xml.html {
head {
title("Folder Comparison")
}
body {
h1("Folder Comparison")
compareFolders(new File(folder1Path), new File(folder2Path), xml)
}
}
}
println "Comparison completed. HTML output file created: ${outputFile.absolutePath}"
```
请将代码中的`/path/to/folder1`和`/path/to/folder2`替换为要比较的实际文件夹路径。执行脚本后,将生成一个名为`output.html`的HTML文件,其中包含文件夹之间的差异信息以HTML表格的形式呈现。
相关推荐
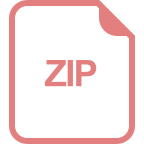
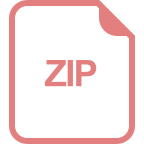
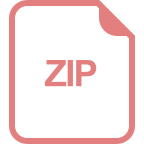






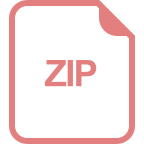
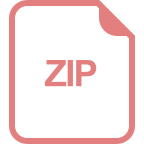
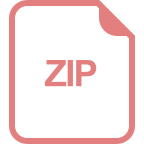
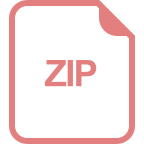
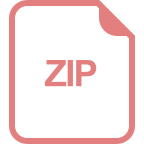
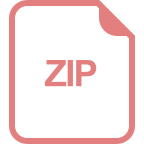
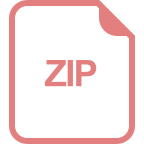