@JsonFormat 处理时间戳
时间: 2024-01-06 16:25:54 浏览: 228
@JsonFormat注解可以帮助我们将时间戳格式化成我们想要的样子。通过在属性上添加@JsonFormat注解,并指定pattern参数来定义时间的格式,可以将时间戳转换为指定格式的字符串。此外,还可以使用timezone参数来指定时区,以确保时间的准确性。
以下是一个示例代码,演示了如何使用@JsonFormat注解处理时间戳:
```java
import com.fasterxml.jackson.annotation.JsonFormat;
public class Example {
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8")
private Date signOutTime;
// getter and setter methods
}
```
在上述示例中,我们在signOutTime属性上添加了@JsonFormat注解,并指定了pattern参数为"yyyy-MM-dd HH:mm:ss",timezone参数为"GMT+8"。这样,在将时间戳转换为字符串时,会按照指定的格式进行格式化,并考虑到指定的时区。
通过使用@JsonFormat注解,我们可以将时间戳转换为我们想要的格式,并将其传递给前端。
相关问题
@JsonFormat秒级时间戳
@JsonFormat可以用于将Date类型的时间转换为指定的格式,其中pattern参数用于指定格式,timezone参数用于指定时区。但是@JsonFormat无法直接将秒级时间戳转换为指定格式的时间。如果要将秒级时间戳转换为指定格式的时间,可以使用自定义的序列化器来实现。首先,创建一个实现JsonSerializer接口的类,重写serialize方法,在该方法中将秒级时间戳转换为指定格式的时间字符串。然后,将该自定义的序列化器应用于需要转换的字段或类上使用@JsonSerialize注解。例如:
```java
public class CustomDateSerializer extends JsonSerializer<Long> {
@Override
public void serialize(Long timestamp, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String formattedDate = sdf.format(new Date(timestamp * 1000));
jsonGenerator.writeString(formattedDate);
}
}
```
```java
public class MyClass {
@JsonSerialize(using = CustomDateSerializer.class)
private Long timestamp;
// other properties
}
```
这样,当使用@JsonFormat注解无法满足需求时,可以使用自定义的序列化器来实现对秒级时间戳的转换。
@JsonFormat 时间戳转date格式化
在Spring Boot项目中,如果你想要将JSON格式中的时间戳转换成Date对象,并进行自定义格式化的设置,可以利用Jackson库提供的`@JsonFormat`注解。这个注解通常放在getter方法上,比如`getCreatedAt()`。
例如:
```java
import com.fasterxml.jackson.annotation.JsonFormat;
public class YourEntity {
private Date createdAt;
// 使用@JsonFormat指定日期格式
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "Asia/Shanghai")
public Date getCreatedAt() {
return createdAt;
}
// 设置方法...
}
```
在这个例子中,`pattern`属性设置了日期时间的格式,如"yyyy-MM-dd HH:mm:ss"代表年月日小时分钟秒;`timezone`属性则指定了时区,这里是"Asia/Shanghai"。当序列化到JSON时,`createdAt`字段会按照这个格式显示,而从JSON反序列化时,Jackson会尝试解析成匹配该格式的Date对象。
阅读全文
相关推荐
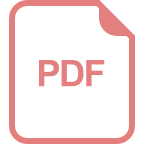
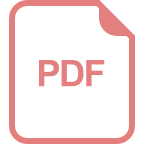
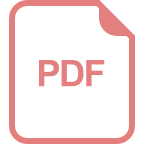













