c++中map的用法
时间: 2023-12-01 09:03:49 浏览: 47
C++中的map是一种关联式容器,它提供了一种将键映射到值的方法。以下是map的用法示例:
1.创建一个map对象并插入键值对:
```c++
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> mp;
mp.insert(make_pair("apple", 3));
mp.insert(make_pair("banana", 2));
mp.insert(make_pair("orange", 4));
return 0;
}
```
2.遍历map并访问键和值:
```c++
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> mp;
mp.insert(make_pair("apple", 3));
mp.insert(make_pair("banana", 2));
mp.insert(make_pair("orange", 4));
for (auto it = mp.begin(); it != mp.end(); it++) {
cout << it->first << " " << it->second << endl;
}
return 0;
}
```
3.使用下标运算符访问map中的值:
```c++
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> mp;
mp.insert(make_pair("apple", 3));
mp.insert(make_pair("banana", 2));
mp.insert(make_pair("orange", 4));
cout << mp["apple"] << endl; // 输出:3
return 0;
}
```
4.删除map中的键值对:
```c++
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> mp;
mp.insert(make_pair("apple", 3));
mp.insert(make_pair("banana", 2));
mp.insert(make_pair("orange", 4));
mp.erase("banana");
for (auto it = mp.begin(); it != mp.end(); it++) {
cout << it->first << " " << it->second << endl;
}
return 0;
}
```
相关推荐
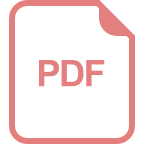
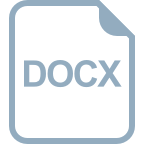













