怎么检查字符串是否包含特定字符或子串?
时间: 2024-12-18 16:30:21 浏览: 49
在Python中,有几种方法可以检查字符串是否包含特定字符或子串:
1. **使用 `in` 关键字**:这是最直观的方式,它会返回一个布尔值,如果子串存在则为 `True`,否则为 `False`。
```python
s = "Hello, World!"
if "World" in s:
print("Substring found.")
```
2. **`str.contains()` 或 `.find()` 方法**:如果你在Python 3.8及以上版本,可以使用 `str.contains()` 方法;而在早期版本中,通常使用 `str.find(substring)`,该方法返回子串首次出现的位置(如果找到),否则返回 `-1`。
```python
if s.find("World") != -1:
print("Substring found at position:", s.find("World"))
```
3. **正则表达式**:如果你需要更复杂的模式匹配,可以使用 `re` 模块的 `search()` 或 `match()` 函数。例如:
```python
import re
pattern = r"\bWorld\b" # 匹配整个单词 "World"
match = re.search(pattern, s)
if match:
print("Substring found.")
```
4. **列表推导式**:如果你想一次性检查多个子串,可以使用列表推导式。
```python
substrings_to_check = ["World", "Python"]
contains_substrings = [substring in s for substring in substrings_to_check]
if any(contains_substrings):
print("At least one substring is found.")
```
阅读全文
相关推荐








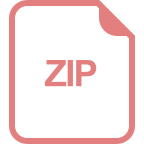









