基于顺序或链式存储结构,使用C++编写程序,实现下述功能: 1、初始化一个空栈 2、如有必要:置空栈、销毁栈、判栈空、判栈满 3、入栈、出栈、取栈顶元素 4、数制转换
时间: 2024-11-04 09:15:07 浏览: 7
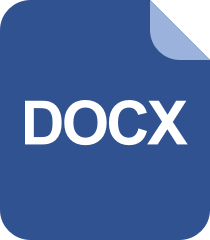
《数据结构C++版》实验一:线性表的顺序存储结构实验报告
为了实现基于顺序(数组)或链式(动态数组或链表)的栈数据结构,并完成给定的功能,我们可以分别针对这两种情况来编写C++代码。这里我将展示如何使用链式存储结构(由于链表操作更直观)来实现这个栈。
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct Node {
int data;
Node* next;
};
// 创建一个空栈
Node* createStack() {
return new Node{0, nullptr};
}
// 判栈是否为空
bool isEmpty(Node* top) {
return top == nullptr;
}
// 判栈是否已满(假设链表的大小有限)
bool isFull(Node** top, int capacity) {
// 对于链表,理论上永远不会"满"
// 如果你想限制容量,可以检查实际元素数量接近capacity
return false;
}
// 入栈操作
void push(Node*& top, int value) {
Node* newNode = new Node{value, top};
top = newNode;
}
// 出栈操作
int pop(Node*& top) {
if (isEmpty(top)) {
throw runtime_error("Stack is empty");
}
int value = top->data;
Node* temp = top;
top = top->next;
delete temp;
return value;
}
// 取栈顶元素(不删除)
int topElement(Node* top) {
if (isEmpty(top)) {
throw runtime_error("Stack is empty");
}
return top->data;
}
// 数制转换示例(这里仅提供一个通用函数模板)
string convertBase(int num, int base) {
if (base <= 0 || base > 36) {
throw invalid_argument("Invalid base; it should be between 2 and 36");
}
string digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
string result = "";
while (num > 0) {
result += digits[num % base];
num /= base;
}
reverse(result.begin(), result.end());
return result;
}
int main() {
Node* stackTop = createStack();
try {
// 使用栈并进行操作
// push(5), push(10), etc.
cout << "Top element before pushing: " << topElement(stackTop) << endl;
push(stackTop, 15);
cout << "After pushing 15: " << topElement(stackTop) << endl;
cout << "Converted to hexadecimal: " << convertBase(pop(stackTop), 16) << endl;
// ...更多操作...
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
// 置空栈
while (!isEmpty(stackTop)) {
pop(stackTop);
}
delete stackTop;
return 0;
}
```
请注意,在实际应用中,如果你需要使用数组实现栈,可以使用固定大小的`vector<int>`替代链表节点。上述链表版本适用于需要动态扩容的情况。
阅读全文
相关推荐
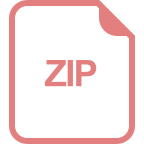
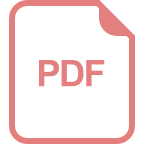
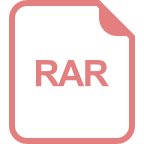
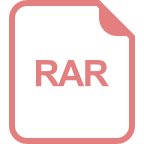
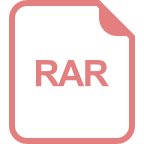
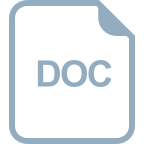
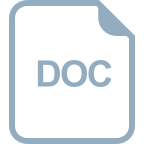
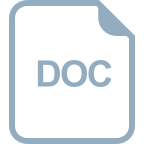
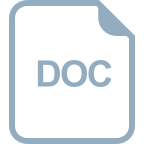
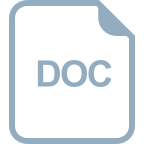
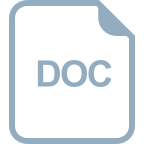
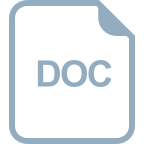
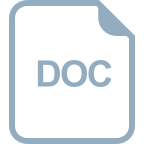
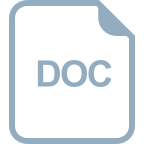
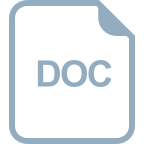
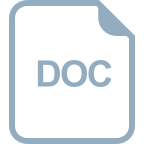
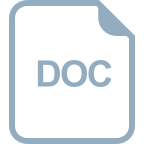
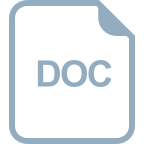