python单词表统计
时间: 2024-07-09 19:01:23 浏览: 38
在Python中,我们可以使用内置模块和第三方库来方便地统计文本(如单词表)中的单词频率。这里是一个基本的方法:
```python
import re
from collections import Counter
def word_count(text):
# 使用正则表达式去除标点符号并分割单词
words = re.findall(r'\b\w+\b', text.lower())
# 使用Counter计算每个单词的出现次数
word_counts = Counter(words)
return word_counts
# 示例用法
text = "这是一段示例文本,包含了多个单词,我们将统计这些单词的频率。"
word_counts_result = word_count(text)
print(word_counts_result)
相关问题
python统计excel中出现次数_使用python&xlrd统计Excel表中特定单词的出现次数
你可以使用 python 的 xlrd 库来读取 Excel 表格数据,然后使用正则表达式来匹配特定单词,并统计出现次数。以下是一个基本的示例代码:
```
import xlrd
import re
# 打开 Excel 文件
workbook = xlrd.open_workbook('example.xlsx')
sheet = workbook.sheet_by_index(0)
# 统计单词出现次数的字典
word_count = {}
# 正则表达式匹配单词
pattern = re.compile(r'\bword\b', re.IGNORECASE)
# 遍历 Excel 表格的每一行,统计单词出现次数
for i in range(sheet.nrows):
row = sheet.row_values(i)
for cell in row:
if isinstance(cell, str):
match = pattern.findall(cell)
if match:
for word in match:
word_count[word] = word_count.get(word, 0) + 1
# 输出单词出现次数
for word, count in word_count.items():
print(f'{word}: {count}')
```
在示例代码中,我们打开了一个名为 `example.xlsx` 的 Excel 文件,并读取了第一个工作表的数据。然后,我们使用正则表达式 `r'\bword\b'` 匹配单词 `word`,并将其出现次数存储在 `word_count` 字典中。最后,我们遍历 `word_count` 字典,并输出每个单词的出现次数。
你可以将示例代码中的 `example.xlsx` 文件和 `word` 单词替换为你需要统计的 Excel 文件和单词。
python词频统计的结果分析
Python词频统计是一种文本分析方法,可以帮助人们了解一个文本中出现频率较高的关键词,从而更好地理解文本中的主题和内容。下面是Python词频统计的结果分析:
1. 词频统计结果显示了文本中出现频率最高的单词。这些单词通常是文本的主题和关键词,可以提供对文本主题的初步认识。
2. 词频统计结果还可以帮助人们了解文本的语言风格。例如,一些文本可能使用了大量的专业术语或者口语表达,这些都可以通过词频统计来了解。
3. 词频统计结果可以用于比较不同文本之间的相似性。如果两个文本中出现频率最高的单词相似,那么这两个文本可能涉及相似的主题或内容。
4. 词频统计还可以用于发现文本中的异常词汇。例如,如果某个单词在文本中出现的频率异常高,那么这个单词可能是一个重要的关键词。
总之,Python词频统计是一种简单而有效的文本分析方法,可以帮助人们更好地理解文本的主题和内容,并发现其中的规律和异常。
相关推荐
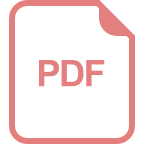
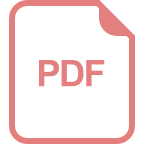
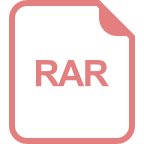












