如何在C语言中编写一个检查字符串是否为全字母句(由大小写字母组成无空格标点符号的句子)的函数?
时间: 2024-12-22 12:27:20 浏览: 1
在C语言中,你可以创建一个函数来检查一个字符串是否仅包含大小写字母,可以采用遍历字符串并逐字符判断其是否属于ASCII码表中的大写字母(A-Z)和小写字母(a-z)。下面是一个简单的函数实现示例:
```c
#include <ctype.h> // 包含ASCII字符测试宏
int is_all_alphabetic(char *str) {
int i = 0;
while (str[i]) { // 遍历直到遇到字符串结束符'\0'
if (!isalpha(str[i])) { // 如果不是字母,返回false
return 0;
}
i++;
}
return 1; // 如果所有字符都是字母,则返回true
}
// 使用函数的例子
int main() {
char str[] = "HelloWorld"; // 测试字符串
if (is_all_alphabetic(str)) {
printf("'%s' is a full alphabet sentence.\n", str);
} else {
printf("'%s' is not a full alphabet sentence.\n", str);
}
return 0;
}
```
相关问题
C语言编程输入一个字符串,统计字母(不分大小写)、数字、其他字符的个数并输出
在C语言编程中,要统计一个字符串中的字母(不分大小写)、数字和其他字符的个数,你可以使用`ctype.h`库中的函数来判断每个字符的类型。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void count_chars(char *str) {
int alpha_count = 0; // 字母计数
int digit_count = 0; // 数字计数
int other_count = 0; // 其他字符计数
for (int i = 0; str[i] != '\0'; i++) { // 遍历字符串
if (isalpha(str[i])) { // 判断是否为字母
alpha_count++;
} else if (isdigit(str[i])) { // 判断是否为数字
digit_count++;
} else { // 其它字符(包括空格、标点等)
other_count++;
}
}
printf("字母数量: %d\n", alpha_count);
printf("数字数量: %d\n", digit_count);
printf("其他字符数量: %d\n", other_count);
}
int main() {
char input[100]; // 输入字符串
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 获取用户输入,并去除换行符
input[strcspn(input, "\n")] = '\0'; // 替换换行符为结束符
count_chars(input); // 调用函数统计字符
return 0;
}
```
当你运行这个程序并输入一个字符串后,它会分别显示字母、数字和其他字符的数量。
对一个字符串中的所有单词,如果单词的首字母不是大写字母,则把单词的首字母变成大写字母。 字符串由单词、空格和英文标点符号组成; 单词由大小写英文字母组成,单词之间通过空格或英文标点分隔。使用C语言实现
可以通过以下步骤实现:
1. 定义一个函数,接收一个字符串作为参数。
2. 使用 strtok 函数将字符串按照空格和标点符号分割成单词。
3. 对于每个单词,判断首字母是否为大写字母。
4. 如果首字母不是大写字母,则将首字母变成大写字母。
5. 将修改后的单词拼接成新的字符串。
6. 返回新的字符串。
下面是具体实现代码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
char* capitalizeWords(char* str) {
char* token = strtok(str, " ,.?!;:");
char* newStr = (char*)malloc(strlen(str) + 1);
strcpy(newStr, "");
while (token != NULL) {
if (!isupper(token[0])) {
token[0] = toupper(token[0]);
}
strcat(newStr, token);
strcat(newStr, " ");
token = strtok(NULL, " ,.?!;:");
}
newStr[strlen(newStr) - 1] = '\0';
return newStr;
}
int main() {
char str[] = "hello, my name is john. what's your name?";
printf("%s\n", capitalizeWords(str));
return 0;
}
```
输出结果为:
```
Hello, My Name Is John. What's Your Name?
```
阅读全文
相关推荐
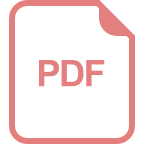
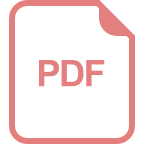
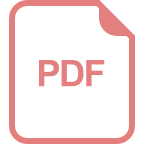













